This a Demo Example AWT (Abstract Window Toolkit) Control in Java.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 | import java.awt.*; import java.awt.event.*; public class AwtControlDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtControlDemo(){ prepareGUI(); } public static void main(String[] args){ AwtControlDemo awtControlDemo = new AwtControlDemo(); awtControlDemo.showEventDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showEventDemo(){ headerLabel.setText("Control in action: Button"); Button okButton = new Button("OK"); Button submitButton = new Button("Submit"); Button cancelButton = new Button("Cancel"); okButton.setActionCommand("OK"); submitButton.setActionCommand("Submit"); cancelButton.setActionCommand("Cancel"); okButton.addActionListener(new ButtonClickListener()); submitButton.addActionListener(new ButtonClickListener()); cancelButton.addActionListener(new ButtonClickListener()); controlPanel.add(okButton); controlPanel.add(submitButton); controlPanel.add(cancelButton); mainFrame.setVisible(true); } private class ButtonClickListener implements ActionListener{ public void actionPerformed(ActionEvent e) { String command = e.getActionCommand(); if( command.equals( "OK" )) { statusLabel.setText("Ok Button clicked."); } else if( command.equals( "Submit" ) ) { statusLabel.setText("Submit Button clicked."); } else { statusLabel.setText("Cancel Button clicked."); } } } } |
The output of the above code.
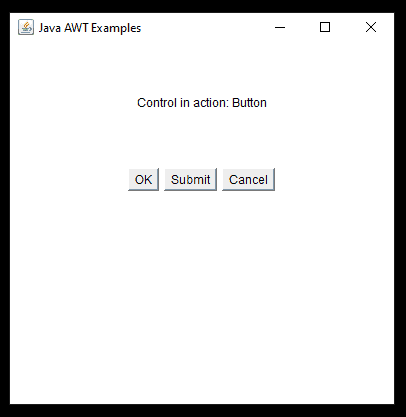