An exception in Java is an event that arises during the execution of a program i.e. during run–time. The occurrence of an exception in a program disrupts the normal flow of instructions. This causes the termination of the program or application and therefore needs to be handled by calling an exception handler, which deals with the exception.
An exception may occur for the invalid user input, or the files that need to be opened cannot be found., or loss of network connection.
Exception handling is a mechanism to maintain the normal flow of a program by handling runtime errors. The main advantage of exception handling is to ensure that even when an exception occurs, the program’s flow doesn’t break.
Type of exception in Java:
- Checked:
Checked Exceptions are also known as compile-time exceptions. These exceptions are checked by the compiler during compile-time. If a checked exception is found within the method then the method must be handled by try and catch or must declare an exception by throws keyword. These exceptions are not to be ignored.
Some of the checked Exceptions occur are SQLException, IOException, ClassNotFoundException, etc. - Unchecked Exception:
Unchecked Exceptions are also known as run-time exceptions. These exceptions occur during the time of execution of a program that is at run-time. These exceptions are not checked during compilation.
Some of the Unchecked Exceptions occur are ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException, etc. - Error:
Errors are not an exception but are the problem that a user or programmer cannot handle. We can say that these are irrecoverable such as VirtualMachineError, OutOfMemoryError, AssertionError, etc.
Exception Hierarchy:
All exception classes are subtypes of the java.lang.Exception class. The exception types and errors are the subclasses of the Throwable class. Furthermore, they are also divided into a runtime exception and other exceptions as shown in the figure.
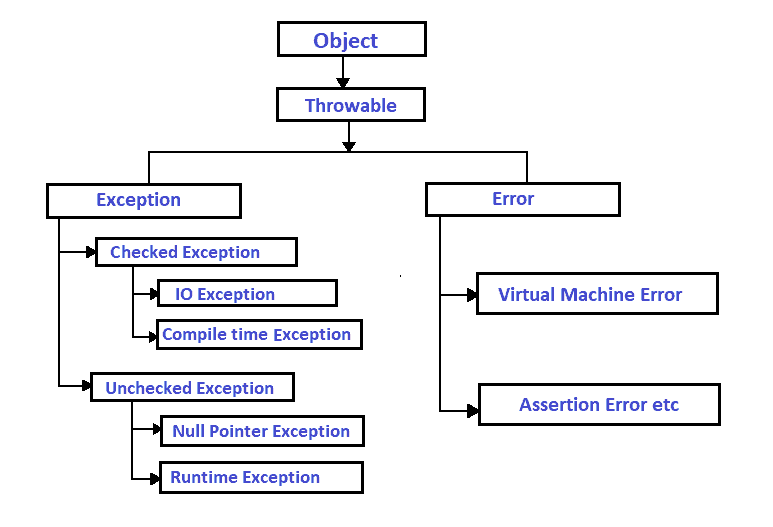
Handling Exception in Java:
Exception in Java is handled using five keywords:
1. try
2. catch
3. throw
4. throws
5. finally.
Try-Catch:
This is one of the common ways to handle the exception. The combination of try and catch keyword is used in a method to catch an exception.
The try block contains the exception code and the catch block is used to handle the exception if it occurs in the try block.
Syntax for try-catch block:
1 2 3 4 5 6 7 8 | try { // Block of exception code } catch(Exception e) { // Block of code to handle exception } |
Example: Java program to demonstrate try-catch:
Let us see it in an example, where the code tries to try to access the fourth element in an array that has only 3 elements:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | public class TryCatchTest { public static void main(String args[]) { try { int a[] = new int[3]; System.out.println("Accessing element four :" + a[4]); } catch (Exception e) { System.out.println("Not Found"); } } } |
Output:
Not Found
Java Multiple Catch Block:
The code having multiple catch blocks which are followed by a try block. If there are different exceptions then each block of multiple catches must contain different exception handlers. Also, one catch block is executed at a time.
Syntax for Multiple Catch Block:
1 2 3 4 5 6 7 8 9 10 | try { // Exception code } catch (ExceptionType1 e1) { // Catch block } catch (ExceptionType2 e2) { // Catch block } catch (ExceptionType3 e3) { // Catch block } |
Example: Java program to demonstrate the Multiple catch block:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | public class MultipleCatchTest { public static void main(String[] args) { try { int a[]=new int[5]; a[5]=30/0; } catch(ArithmeticException e) { System.out.println("Arithmetic Exception occurs"); } catch(ArrayIndexOutOfBoundsException e) { System.out.println("ArrayIndexOutOfBounds Exception occurs"); } } } |
Output:
Arithmetic Exception occurs
Throw:
The throw keyword in Java is used to explicitly throw an exception from any block of code. throw keyword allows us to create a custom error or throw a custom exception.
Syntax of throw keyword:
1 | throw new exception_type("error message"); |
For example, we can throw ArithmeticException when we divide the number by 3, or any other numbers, we only need to do is set the condition and throw an exception using throw keyword. Such as:
1 | throw new ArithmeticException("cannot divide a number by 3"); |
Example: Java Program to demonstrate throw:
Let us see it in a program:
Check for roll no. greater than 12, if true then allowed and if not then not allowed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | public class ThrowTest { static void checkRollNo(int roll) { if (roll < 12) { throw new ArithmeticException("Not Allowed to Enter."); } else { System.out.println("Allowed to Enter"); } } public static void main(String[] args) { checkRollNo(10); } } |
Output:
1 2 3 | Exception in thread "main" java.lang.ArithmeticException: Not Allowed to Enter. at ThrowTest.checkRollNo(ThrowTest.java:7) at ThrowTest.main(ThrowTest.java:16) |
Throws
throws keyword in java is used to declare an exception. It indicates that the method may throw an exception and it’s better to handle the exception using a try-catch block to maintain the normal flow of the program.
Syntax of throws keyword:
1 2 3 4 | return_type method_name() throws exception_class_name { //method code } |
Example: Java Program to demonstrate throws:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | import java.io.IOException; public class ThrowsTest { void method1()throws IOException { throw new IOException("Error");//checked exception } void method2()throws IOException { method1(); } void method3() { try{ method2(); }catch(Exception e) { System.out.println("exception is handled"); } } public static void main(String args[]) { ThrowsTest obj=new ThrowsTest(); obj.method3(); System.out.println("normal flow maintained"); } } |
Output:
exception is handled normal flow maintained
Finally
Finally block is used to execute the code followed by the try-catch block. finally block is always executed whether an exception is handled or not. This block appears at the end of the catch block.
Syntax of throws keyword:
1 2 3 4 5 6 7 8 9 10 11 | try { // Protected code } catch (Exception1 e1) { // Catch block } catch (Exception2 e2) { // Catch block } catch (Exception3 e3) { // Catch block }finally { // The finally block is always executed. } |
Example: Java Program to demonstrate finally:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | public class FinallyTest { public static void main(String args[]) { int x[] = new int[5]; try { System.out.println("Access element 6 :" + x[6]); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Exception thrown :" + e); }finally { x[3] = 4; System.out.println("Value of third element: " + x[3]); System.out.println("Execution of finally."); } } } |
Output:
Exception thrown :java.lang.ArrayIndexOutOfBoundsException: 6
Value of third element: 4
Execution of finally.