This post in queue program in java using array covers insertion, deletion, and display process in queue.
A queue is a special type of collection created to store the elements in FIFO(first-in-first-out) order.
It is used to hold the elements before processing it.
Implementing Queue using Array is simple where we create an array of size n. We also take two variables for the front and rear end. Both will be initialized to 0. The rear is the end of the index element and the front is the index of first element. As it follows FIFO The first element inserted will be deleted first.
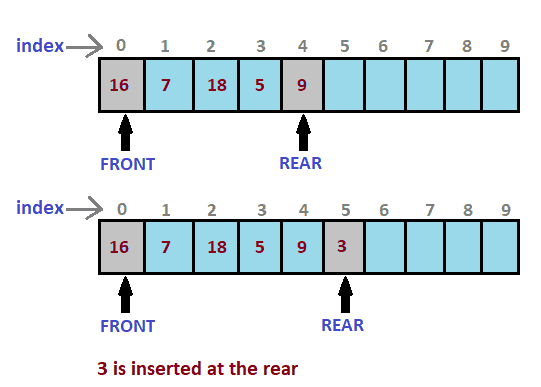
Java program on queue using array
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | import java.io.*; class QueueJava { static int i, front, rear, item, max=5,ch; static int ar[]=new int[5]; QueueJava() { front = -1; rear = -1; } public static void main(String args[])throws IOException { while((boolean)true) { System.out.println("Choose Options"); System.out.println("1. To insert"); System.out.println("2. To delete"); System.out.println("3. To display"); System.out.println("4. To Exit"); BufferedReader br=new BufferedReader(new InputStreamReader(System.in)); ch=Integer.parseInt(br.readLine()); switch(ch) { //Insertion case 1: if(rear>=max) { System.out.println("Queue is Full"); } else { BufferedReader b = new BufferedReader(new InputStreamReader(System.in)); System.out.println("Enter the Element: "); item = Integer.parseInt(b.readLine()); rear=rear+1; ar[rear]=item; } break;//End of Insertion //Deletion case 2: if(front == -1) { System.out.println("Queue is Empty"); } else { front = front+1; item = ar[front]; System.out.println("Deleted Item: "+item); } break;//End of Deletion //Display case 3: System.out.println("Elements in the Queue are:"); for(int i = front+1; i <= rear; i++) { System.out.println(ar[i]); } break;//End of Display case 4: break; } } } } |
Output Queue using Arrays:
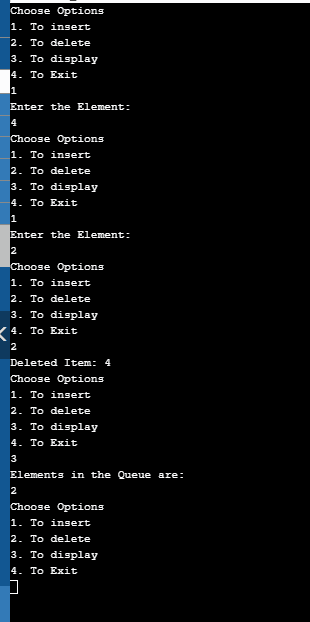