In this another java tutorial lesson on Java alphabetical sort, we will learn how to sort strings in an order of alphabets.
Let’s begin.
Program explanation for arranging string in alphabetic order in java:
First, we will take the user input for how many strings to enter? with the help scanner class. Then ask the user to input all the strings one by one and get them with help of for loop.
Then to sort strings that are stored in a string array, we compare the first alphabet of each array to display them in alphabetic order in java with the help of the compareTo() function. If the first alphabet is the same it will compare the second alphabet and so on.
Java Program to Sort String in an Alphabetical Order
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | //java sort string import java.util.Scanner; public class JavaSortString { public static void main(String[] args) { int lenStr; String temp; Scanner scan = new Scanner(System.in); //number of strings System.out.print("How many Strings would you like to enter? "); lenStr = scan.nextInt(); String str[] = new String[lenStr]; Scanner scan2 = new Scanner(System.in); //getting all the strings System.out.println("Enter the " + lenStr + " Strings: "); for (int i = 0; i < lenStr; i++) { str[i] = scan2.nextLine(); } scan.close(); scan2.close(); //Sorting strings with compareTo() for (int i = 0; i < lenStr; i++) { for (int j = i + 1; j < lenStr; j++) { if (str[i].compareTo(str[j]) > 0) { temp = str[i]; str[i] = str[j]; str[j] = temp; } } } //Print the sorted strings System.out.print("Strings sorted n alphabetical order: "); for (int i = 0; i <= lenStr - 1; i++) { System.out.print(str[i] + ", "); } } } |
Output: After executing the above program, the following output will be displayed.
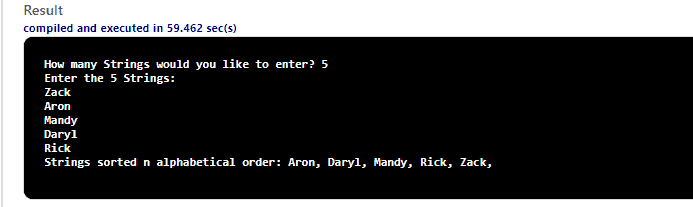