White Space removal program in java:
In this post, we will learn how to remove white spaces from a string in java. We will learn the two ways to remove white spaces in java.
First by using in-built methods called replaceAll() and second without the use of in-built methods (for loop).
1. Java program to remove white spaces in a string using replaceAll().
This is the easiest method to remove spaces from a string in java. replaceAll() takes in two parameters, one is the string to be replaced and another one is the string to be replaced with.
In this example, we will take the string from the user input with the help of a scanner class and as a parameter, we pass “\\s” that will be replaced with “”.
Example Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | import java.util.Scanner; public class WhiteSpaceRemoval { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Enter the string with spaces"); String str1 = sc.nextLine(); //relaceAll() String str2 = str1.replaceAll("\\s", ""); System.out.println(str2); } } |
The output of removing spaces from a string in java:
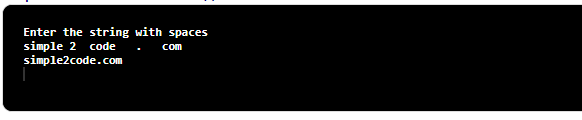
2. Java program to remove white spaces in a string using for loop
This is another method to remove white spaces. Although the above approach is recommended when it comes to creating a real-time application. This is mostly for interview programs asked.
In this method, we take the user input for string and convert them to character Array(charArray) and check those characters for white spaces by running it into the iteration. for loop will iterate until the length of the entered string.
Example Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | import java.util.Scanner; public class WhiteSpaceRemoval { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Enter the string with spaces"); String inputString = sc.nextLine(); char[] charArray = inputString.toCharArray(); String resultString = ""; for (int i = 0; i < charArray.length; i++) { if ((charArray[i] != ' ') && (charArray[i] != '\t')) { resultString = resultString + charArray[i]; } } System.out.println("Result String without spaces: " + stringWithoutSpaces); sc.close(); } } |
The output of removing spaces from a string in java without replaceAll():
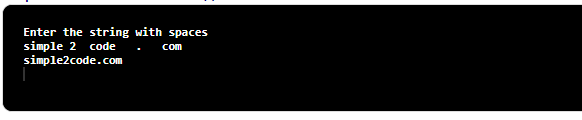