This post shows how to find a square root of a number in java. We will learn two methods to find the square root one by an in-built math method called Math.sqrt() and the other by using do-while loop.
let’s begin.
1. Program to find square root using Math.sqrt() in java
This is a simple and easy method we only need to use Math.sqrt() which returns the square root value when an argument of double is passed to it. For positive or negative zero arguments, it returns the same value as that of the argument.
Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | import java.util.Scanner; public class SquareRootjava { public static void main(String args[]) { Scanner sc = new Scanner(System.in); //user input System.out.println("Enter the number"); double number = sc.nextDouble(); //using method to get square root double squareRoot = Math.sqrt(number); //Display System.out.print("Square root of " + number + " is " + squareRoot); } } |
The output for the square root using Math.Sqrt:
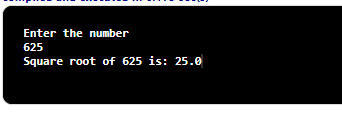
2. Program to find square root using do-while in java
This is another method to find the square root without using Math.sqrt() in java. Here we used the so-while loop. We take the user input passed to squareRootResult() method and run the do-while loop. After the calculation, we return the result back and display it as shown in the program below.
Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | import java.util.Scanner; public class SquareRoot { public static double squareRootResult(int n) { double temp; double returnResult = n / 2; do { temp = returnResult; returnResult = (temp + (n / temp)) / 2; } while ((temp - returnResult) != 0); return returnResult; } public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Enter the number"); int number = sc.nextInt(); System.out.print("Square root of " + number + " is: " + squareRootResult(number)); sc.close(); } } |
Output:
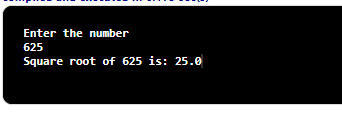