This article will show you how to print a simple pyramid pattern of stars in java.
Patterns are one of the easiest ways to improve the coding skills for java. The frequent use of loops can increase your skills and printing pattern order.
We will see two programs:
- To print a full pyramid by declaring the number of rows in a program.
- To print a full pyramid by taking user input for the number of rows.
1. Java program to print the full pyramid pattern.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | //Pattern full pyramid public class Main { public static void main(String[] args) { int rows = 6; for (int i = 0; i < rows + 1; i++) { for (int j = rows; j > i; j--) { System.out.print(" "); } for (int k = 0; k < (2 * i - 1); k++) { System.out.print("*"); } System.out.println(); } } } |
After the execution of the program, you will get the following result:
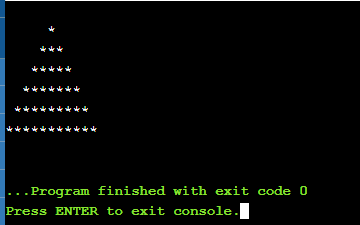
2. Java program to print the full pyramid pattern (user input)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i = 0; i < rows + 1; i++) { for (int j = rows; j > i; j--) { System.out.print(" "); } for (int k = 0; k < (2 * i - 1); k++) { System.out.print("*"); } System.out.println(); } } } |
After the execution of the program, you will get the following result:
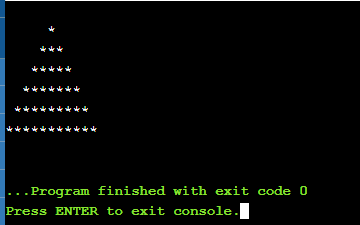