This is a tutorial on how to write a java program to display the second largest element in an array.
Find the second-largest element in an array:
In the below program, we take the user input for How many elements does a user wants in an array and what are the elements with the help of the Scanner class. And then declare an integer variable named “Highest” and “secondHighest” which are set to zero.
Now using for loop and if-else if statement, we check for the highest and the second-highest. That is when the 0th index is greater than the largest, we assigned highest to secondHighest and the element at the 0th index to highest. But if the 0th index is larger than the secondHighest then we assigned the 0th index element to secondHighest.
The for loop iteration will keep repeating until the length of an array (that is the number of elements). After the end of for loop, we display the result for the highest and second-highest by printing it.
Java Program for the second-highest element in java with output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | import java.util.Scanner; public class SecondHighestelements { public static void main(String[] args) { int num; int Highest = 0; int secondHighest = 0; Scanner elem = new Scanner(System.in); Scanner sc = new Scanner(System.in); //Number of elements for an Array System.out.println("Enter the number of elements you want:"); num = elem.nextInt(); int[] arr = new int[num]; //User Input for elements System.out.println("Enter the elements in an array:"); for (int i=0; i < num; i++) { arr[i] = sc.nextInt(); } for (int i = 0; i < arr.length; i++) { if (arr[i] > Highest) { secondHighest = Highest; Highest = arr[i]; } else if (arr[i] > secondHighest) { secondHighest = arr[i]; } } //Display Result System.out.println("\nSecond largest number in an entered Array: " + secondHighest); System.out.println("Largest Number in an entered Array: " + Highest); } } |
The Output of the second largest element in an array in java:
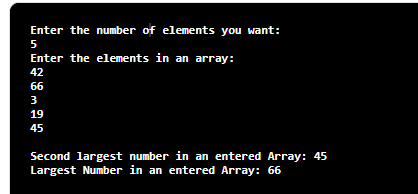