Inheritance is an important pillar for the foundation of Object-Oriented Programming (OOP). It is a process in C# through which one class can acquire the fields and methods of another class.
Inheritance allows us to inherit or acquire the features of the parent class to the child class so that we can be reuse, extend or modify the attributes and behaviour of another class.
Importance of Inheritance:
- For Code Reusability.
- For Method Overriding (to achieve runtime polymorphism).
Important terms related to inheritance.
- Super Class: The class whose properties and functionalities are inherited by the Subclass is known as superclass(a parent class or a base class).
- Sub Class: The class that inherits the property and behavior of the other class(Superclass) is known as subclass( a derived class, extended class, or child class). The subclass can have its own fields and methods along with the fields and methods of the superclass.
Syntax to use inheritance in C#
1 2 3 4 5 | class derived-className : base-className { //fields //methods } |
Types of Inheritance in C#
Below are the various types of inheritance supported in C#.
1. Single Inheritance
It refers to an inheritance where a child inherits a single-parent class. In the diagram below, class B inherits class A.
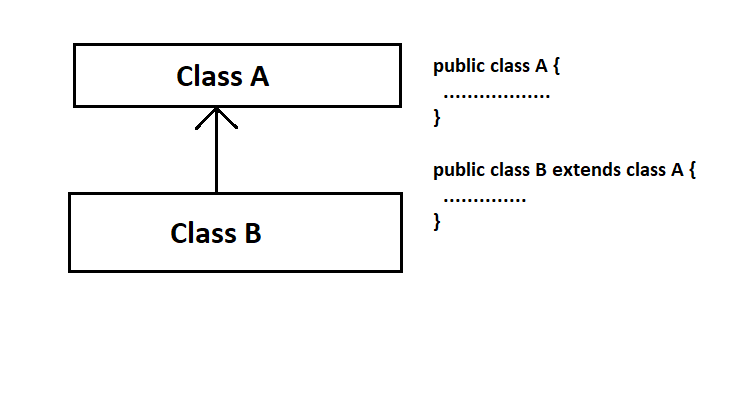
Syntax:
1 2 3 4 | class sub_class_name : base_class_name { //body of subclass }; |
Example: C# example for single inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | using System; namespace InheritanceEg { public class ParentClass { public void printParent() { Console.WriteLine("This is Parent Class"); } } public class ChildClass: ParentClass { public void printChild() { Console.WriteLine("This is Child Class"); } } public class Program { public static void Main(string[] args) { ChildClass obj = new ChildClass(); obj.printParent(); obj.printChild(); } } } |
//Output:This is Parent Class
This is Child Class
2. Hierarchical Inheritance
The process of deriving more than one class from a base class is called hierarchical inheritance. In other words, we create more than one derived class from a single base class as shown in the diagram below.
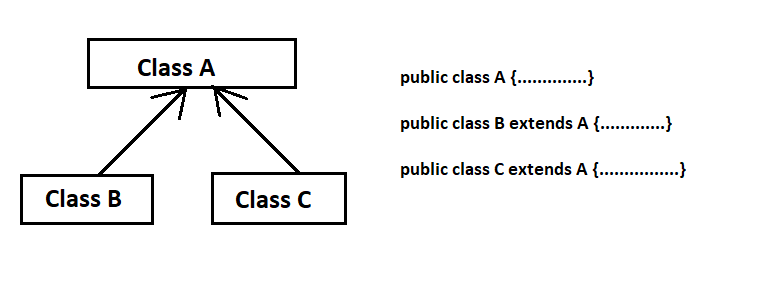
Syntax:
1 2 3 4 5 6 7 8 9 10 11 12 | class One { // Class One members } class Two : One { // Class Two members. } class Three : One { // Class Three members } |
Example: C# example for hierarchical Inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | using System; namespace InheritanceEg { public class ParentClass { public void printParent() { Console.WriteLine("This is Parent Class"); } } public class Son: ParentClass //Inheriting ParentClass { public void printSon() { Console.WriteLine("This is Son's Class"); } } public class Daughter: ParentClass //Inheriting ParentClass { public void printDaughter() { Console.WriteLine("This is Daughter's Class"); } } //Main Class public class Program { public static void Main(string[] args) { Son s = new Son(); Daughter d = new Daughter(); s.printSon(); s.printParent(); d.printDaughter(); d.printParent(); } } } |
//OutputThis is Son's Class
This is Parent Class
This is Daughter's Class
This is Parent Class
3. Multilevel Inheritance
In this type inheritance derived class is created from another derived class. In multilevel inheritance, one class inherits from another class which is further inherited by another class. The last derived class possesses all the members of the above base classes.
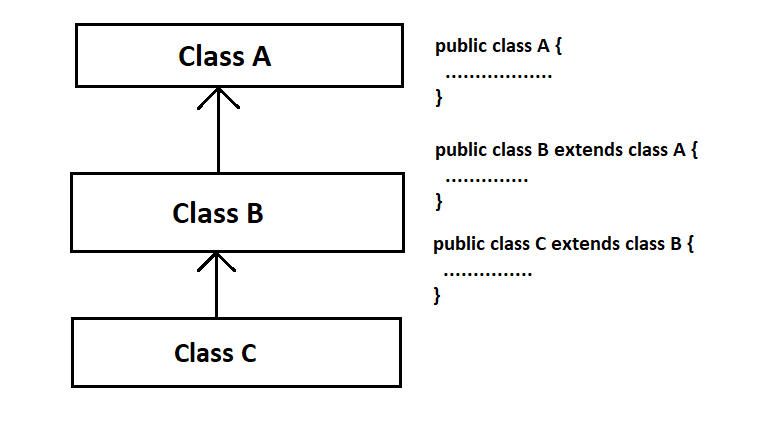
Example: C# example for multilevel inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | using System; namespace InheritanceEg { public class Animal { public void sleep() { Console.WriteLine("Animal sleeps"); } } public class Dog: Animal //Inheriting Animal { public void sound() { Console.WriteLine("Dog barks"); } } public class Puppy: Dog //Inheriting Dog { public void weep() { Console.WriteLine("Puppy weeps"); } } public class Program { public static void Main(string[] args) { Puppy p = new Puppy(); p.sleep(); p.sound(); p.weep(); } } } |
//OutputAnimal sleeps
Dog barks
Puppy weeps
4. Multiple Inheritance (through Interface)
Multiple inheritance is the process where the single class inherits the properties from two or more classes.
In the diagram below, class C inherits from both Class A and Class B.
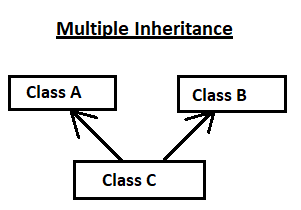
Although, C# does not support multiple inheritance. So we need to use interfaces in C# to use the multiple inheritance. We need to declare the parent class with the interface keyword and the child class with the class keyword.
Example: C# example for multiple inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | using System; namespace InheritanceEg { interface Dog { void dogFunc(); } interface Cat { void catFunc(); } public class Animal: Dog, Cat //Inheriting Dog and Cat interface { public void dogFunc() { Console.WriteLine("I am a Dog"); } public void catFunc() { Console.WriteLine("I am A Cat"); } public void animalFunc() { Console.WriteLine("All are Animals"); } } public class Program { public static void Main(string[] args) { Animal obj = new Animal(); obj.animalFunc(); obj.dogFunc(); obj.catFunc(); } } } |
All are Animals
I am a Dog
I am A Cat