In this tutorial, you will write Polymorphism programs in C#. The program demonstrates for three different data types.
Question:
Write a C# Program to illustrate the use of Polymorphism.
Solution:
In the following program, we create an object for Polymorphism Class, and under this Class, we create three functions of the same name print but varying in argument data types. Now we call the print function by passing the three different values(different data types). We see that despite having the same function name(print), we can still print for three different data types as shown in the program.
C# Program using Polymorphism
Source code: C# polymorphism program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | using System; class Polymorphism { void print(int i) { Console.WriteLine("Printing int: {0}", i); } void print(double f) { Console.WriteLine("Printing float: {0}", f); } void print(string str) { Console.WriteLine("Printing string: {0}", str); } static void Main(string[] args) { Polymorphism p = new Polymorphism(); //Call print to print integer p.print(5); //Call print to print float p.print(500.263); //Call print to print string p.print("Hello World"); } } |
Output:
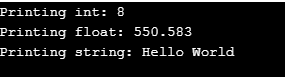