As we know the variables holds the data and the type of data that it holds refers to the data-types. Theses types are: integer, float, double, boolean, etc. and each of this data-types has a specific size such as integer has the memory size of 4 byte and so on.
Now, C# has categorized its data-types in three different parts:
- Value types: int, float, short, char, etc.
- Reference types: String, Class, Object and Interface
- Pointer types: simply Pointers
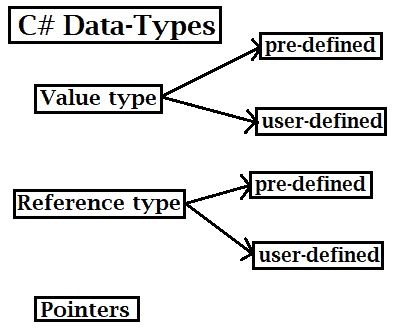
C# contains two general categories of built-in data types: value types and reference types.
The difference between the two types is what a variable contains.
- For a value type, a variable holds an actual value, such 3.1416 or 212.
- For a reference type, a variable holds a reference to the value.
Let us discuss each of them:
1. Value types
Value types are derived from the class System.ValueType. They refer to the direct value used in the program such as integers, float, double and more. These data-types are both integer-based and floating-point types. Memory allocates for the memory space whenever some value type is assigned.
Value types are of two types, Predefined Data Types (Integer, Boolean, etc) and User-defined Data Types (Structure, Enumerations, etc).
Follow the table below for the value type list in C#:
Data Types | Memory Size | Range |
---|---|---|
int | 4 byte | -2,147,483,648 to -2,147,483,647 |
float | 4 byte | 1.5 * 10-45 – 3.4 * 1038, 7-digit precision |
short | 2 byte | -32,768 to 32,767 |
char | 1 byte | -128 to 127 |
long | 8 byte | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
double | 8 byte | 5.0 * 10-324 – 1.7 * 10308, 15-digit precision |
signed short | 2 byte | -32,768 to 32,767 |
unsigned short | 2 byte | 0 to 65,535 |
signed int | 4 byte | -2,147,483,648 to -2,147,483,647 |
unsigned int | 4 byte | 0 to 4,294,967,295 |
signed long | 8 byte | ?9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
unsigned long | 8 byte | 0 – 18,446,744,073,709,551,615 |
decimal | 16 byte | at least -7.9 * 10?28 – 7.9 * 1028, with at least 28-digit precision |
Memory size changes according to the Operating System.
2. Reference Types
Reference types are a bit different than Value Types, this kind of data-types do not contain the actual data stored in a variable, but they contain a reference to the variables.
Reference types specify to the memory location and if the data in the memory location is changed by one of the variables, the other variable automatically reflects this change in value.
They are also of two types, Predefined Data Types (Objects, String and Dynamic) and User-defined Data Types (Classes, Interface).
3. Pointer Type
It is also known as the indicator as it points to the address of a value. Pointer type variables store the memory address of another type. The abilities to do the task is same as the pointers that we use in C or C++.
Syntax(declaration):
It is declare using a * (asterisk symbol).
1 2 3 4 5 | data-type* identifier; //example int* ptr1; char* ptr2; |
Literals
Literals refer to fixed values that are represented in their human-readable form. For the most part, literals and their usage are so intuitive that they have been used in one form or another by all the preceding sample programs.
For example, the number 100 is a literal.
C# literals can be of any simple type. The way each literal is represented depends upon its type.
- To specify a float literal, append an F or f to the constant. For example, 10.19F is of type float.
- To specify a decimal literal, follow its value with an m or M. For example, 9.95M is a decimal literal.
Literals can be any of the following types that can be used in a program:
- Integer Literals
- Floating-point Literals
- Character Literals
- String Literals
- Null Literals
- Boolean Literals
Integer Literals
An integer literal can be decimal(base 10) or hexadecimal(base 16) constant or Octal(base 8). A prefix specifies the base or radix: 0x or 0X for hexadecimal, and there is no prefix id for decimal.
For unsigned and signed, the suffix U and L are used. And the only rule is you can represent these suffix either in uppercase or the lower case and in any order you may prefer.
Example of integer literals:
1 2 3 | 20 // simply int 30u // u for unsigned int 30L // l for long |
Floating-Point Literals
Literals is called the floating-point literals if it has the following part:
- an integer part
- a decimal point
- a fractional part
- an exponent part
We can constitute them in decimal or exponential form. Representation of decimal form required the decimal point or the exponent or the both while the exponential form required the integer part or the fractional part or both.
By default, the floating-point literals are of double type and assigning it directly to a float variable is not possible. Instead, we can use the suffix for a float that is I or F.
Some example to show how can it be used and how it can’t be used in a program:
1 2 3 4 | 4.25671 //valid 254632E-5F //valid 120E //Invalid: missing exponent 240f //Invalid: missing exponent or decimal |
Character Literals:
Character literals are those literals that are enclosed within the single quote(‘ ‘) such as ‘S’ is stored as a character. we can represent the character literals in several ways:
- Single quote: These are the plain character enclosed within a single quote. Eg:
char c = 'S';
- Unicode Representation: These are the universal character denoted in Unicode such as
char c = '\u0061';
//this Unicode value represents a character. - Escape Sequence: This is also a character literal that can be used as a character such as
char c = '\t';
The following table shows some escape sequence that are used in a program with their meaning. Go through them carefully.
Escape sequence | Meaning |
---|---|
\\ | \ character |
\’ | ‘ character |
\” | ” character |
\? | ? character |
\a | Alert or bell |
\b | Backspace |
\f | Form feed |
\n | Newline |
\r | Carriage return |
\t | Horizontal tab |
\v | Vertical tab |
\xhh . . . | Hexadecimal number of one or more digits |
String Literals
This type of literals is enclosed within double-quotes (“”) or with @””. A string also enclosed the same character as the character literal does such as plain character, universal characters and more.
The below example show how the string literals are represented in a program.
1 2 3 4 | "simple, code" "simple, \code" "simple, " "d" "code" @"simple code" |
Boolean Literals
This literal has only two values that show the result and they are true and false. You can assign the value at the beginning while declaring the boo as shown in an example below.
1 2 | bool a = true; bool b = false |