The goto statement is a jump statement that allows the user in the program to jump the execution control to the other part of the program. It jumps to the position where the labeled statement present in the program. The label (tag) is used to spot the jump statement.
NOTE: Remember the use of goto is avoided in programming language because it makes it difficult to trace the control flow of a program, making the program hard to understand and hard to modify.
goto statement Flowchart:
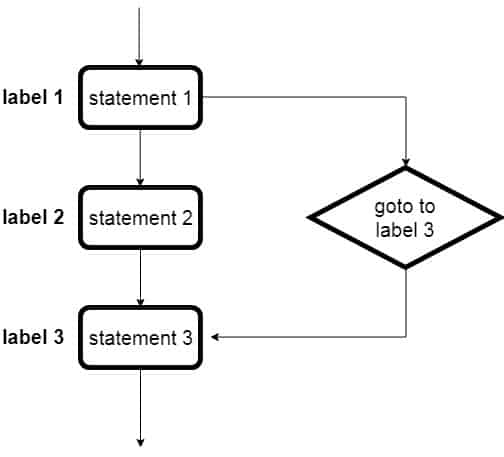
Syntax of goto statement in C++
1 2 3 4 5 6 7 | goto label; .... .... label: statement; //label to jump .... .... statements |
Example of C++ goto statement
The simple example of goto
statement in C++ programming.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include <iostream> using namespace std; int main() { //this is the label ineligible_label: int age; cout << "Enter your age: "; cin >> age; if (age < 18) { cout << "You are below 18 and not eligible to vote.\n"; goto ineligible_label; } else { cout << "You are above 18 and eligible to vote."; } } |
Output:
1 2 3 4 | Enter your age: 16 You are below 18 and not eligible to vote. Enter your age: 21 You are above 18 and eligible to vote. |
Avoid the use of goto
: However, the use of goto
is considered a harmful construct and a bad programming practice in a C++ programming. It makes the program complex and tangled, instead you can achieve the task of goto by using the break and continue statement.