If you want to change the execution from the normal sequence or if you want the program to exit loops, for these purposes you need to use loop control statements.
Loop control statements are also known as Jump statements. It allows you to jump from the loops or if you wish to repeat the loops after some condition is satisfied, jump statements helps you to achieve that.
C++ supports the following control statements.
- break statement
- continue statement
- goto
break statement in C++
break statement terminates the loop and transfers the execution process immediately to a statement following the loop.
break statement is mostly used in a switch statement to terminate the cases present in a switch statement.
The use of break statements in nested loops terminates the inner loop and the control is transferred to the outer loop.
break statement Flowchart:
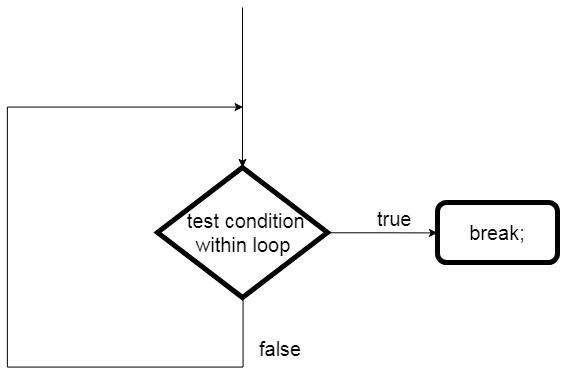
Syntax of break statement in C++:
1 | break; |
Click here for example of break
statement in C++.
continue statement in C++
The continue statement works like a break statement, instead of terminating the loop the continue statement skips the code in between and passes the execution to the next iteration in the loop.
In case of for
loop, the continue statement causes the program to jump and pass the execution to the condition and update expression by skipping further statement. Whereas in the case of while and do…while loops, the continue statement passes the control to the conditional checking expression.
continue statement Flowchart:
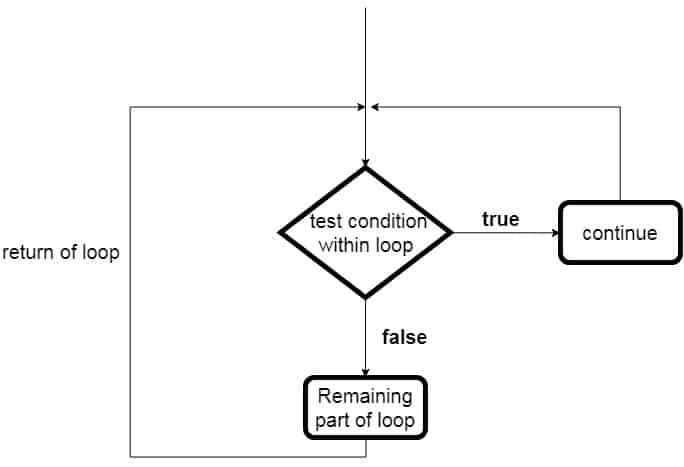
Syntax of continue statement in C++:
1 | continue; |
Click here for example of continue
statement in C++.
goto statement in C++
The goto statement is a jump statement that allows the user in the program to jump the execution control to the other part of the program. It jumps to the position where the labeled statement present in the program. The label (tag) is used to spot the jump statement.
NOTE: Remember the use of goto is avoided in programming language because it makes it difficult to trace the control flow of a program, making the program hard to understand and hard to modify.
goto statement Flowchart:
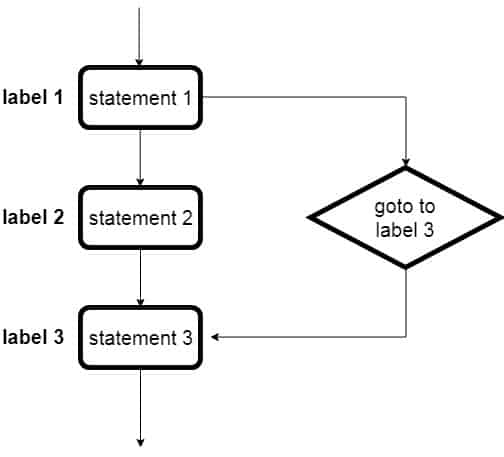
Syntax of goto statement in C++
1 2 3 4 5 6 7 | goto label; .... .... label: statement; //label to jump .... .... statements |
Click here for example of goto
statement in C++.