An exception is an event or an error that arises during the execution of a program i.e. during run–time. The occurrence of an exception in a program disrupts the normal flow of instructions.
So to handle such errors, we use exception handlers. If we do not handle the exception properly then the program may terminate. We can catch an exception through exception handling and our program will not completely terminate.
The main advantage of exception handling is to ensure that even when an exception occurs, the program’s flow doesn’t break.
C++ Exception Handling Keywords
C++ provides three keywords to perform exception handling. They are:
- try: The try block identifies the block of code for which the exception will be activated. It is followed by a catch block.
- catch: The catch indicates the cathching of an exception where you want to catch the error accuring in the program.
- throw: throw keyword is used to throw an exception when a problem arises in a program.
try/catch in C++
Assume that the block of code will cause an error and you want to catch that exception. The try and catch block will help you to do that. You place a code inside the try block which you think causing the problem and catch the statement to catch the exception.
The syntax for try and catch:
1 2 3 4 5 6 7 8 9 10 | try { // Exception code } catch (ExceptionType1 e1) { // Catch block } catch (ExceptionType2 e2) { // Catch block } catch (ExceptionType3 e3) { // Catch block } |
We can place a multiple catch statement on different types of exceptions that may arise by try block code.
Example: C++ try/catch
Use of try, catch and throw keywords in a C++ program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | #include <iostream> using namespace std; double division(int x, int y) { if (y == 0) throw "Division by Zero attempted."; return (x / y); } int main() { int a = 11; int b = 0; double c = 0; try { c = division(a, b); cout << c << endl; } catch (const char *ex) { cerr << ex << endl; } return 0; } |
Output:
Division by Zero attempted.
C++ Standard Exceptions
C++ provides a list of standard exceptions defined in <exception> class that we can use in a program.
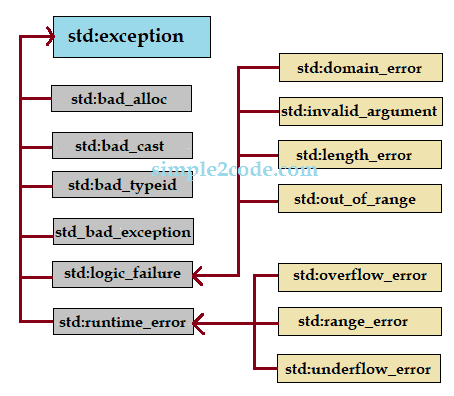
These exceptions and descriptions are listed in the table below.
Exception | Description |
std::exception | This is an exception and the parent class of all standard C++ exceptions. |
std::bad_alloc | This can be thrown by a keyword new. |
std::bad_cast | This can be thrown by dynamic_cast. |
std::bad_exception | A useful device for handling unexpected exceptions in C++ programs. |
std::bad_typeid | An exception thrown by typeid. |
std::logic_error | This exception is theoretically detectable by reading code. |
std::domain_error | This is an exception thrown after using a mathematically invalid domain. |
std::invalid_argument | This exception is thrown for using invalid arguments. |
std::length_error | This exception is thrown after creating a big std::string. |
std::out_of_range | Thrown by at method. |
std::runtime_error | This is an exception that cannot be detected via reading the code. |
std::overflow_error | This exception is thrown after the occurrence of a mathematical overflow. |
std::range_error | This exception is thrown when you attempt to store an out-of-range value. |
std::underflow_error | This exception is thrown after the occurrence of mathematical underflow. |
User-Defined Exceptions
The C++ std::exception class allows us to define objects that can be thrown as exceptions. This new exception can be defined by overriding and inheriting the exception class functionality. This class has been defined in the <exception> header.
Example: user-defined exception in c++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | #include <iostream> #include <exception> using namespace std; class ExceptionEg: public exception { virtual const char *what() const throw () { return "Occurance of new exception"; } }; int main() { ExceptionEg newEx; try { throw newEx; } catch (exception & ex) { cout << ex.what() << '\n'; } return 0; } |
Output:
Occurance of new exception