A string is a sequence of characters that is an object of std::string class in C++. Memory to a string is allocated dynamically that is we can assign memory during runtime as required. Various operations are performed on a string such as concatenation, comparison, conversion, etc.
C++ supports two types of string in a program.
- C-style character string.
- String class type (Standard C++ Library).
C-style character string
C-style was introduced in the C programming language and is continued to be supported by C++. These one-dimensional strings are stored in a series in an array of type char and are terminated by a null character ‘\0’.
Memory presentation of the character array in C/C++:
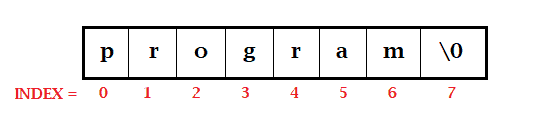
Declaring C-style string:
char name[5] = {'A', 'l', 'e', 'x', '\0'};
The above character for a string ‘Alex‘. But notice that the number f character is 4 but we declare the size 5 as because the last space is reserved for the null character in order to terminate the string.
Although, we can also initialize the above in the following way.
char name[] = "John";
Note that here, we do not have to add a null character at the end. The C++ compiler will automatically place the ‘\0‘ at the end of the string.
Example: C++ program to read and display the string
1 2 3 4 5 6 7 8 9 10 11 12 13 | #include <iostream> using namespace std; int main() { char str[100]; cout << "Enter a string: "; cin.get(str, 100); cout << "Entered String is: " << str << endl; return 0; } |
Enter a string: This is simple2code.com
Entered String is: This is simple2code.com
Notice that we use cin.get
in order to get the string from the user that contains white spaces in a string. It takes two argument name of a string and the size of an array.
String class type
The standard C++ library provides a string class that supports the various operation on a string. It is written as std::string.
Also, instead of char type, we create a string object to hold the string. It does not require fined length like in char type, we can extend the character according to our need.
Now in order to use the class to perform various operations on a string, we need to include the following header file.
#include<cstring>
Example: C++ program to read and display the string using string object
Before performing on class type with some operation, let us first see a simple example of string using its object.
1 2 3 4 5 6 7 8 9 10 11 12 13 | #include <iostream> using namespace std; int main() { string str; cout << "Enter a string: "; getline(cin, str); cout << "Entered String is: " << str << endl; return 0; } |
Enter a string: This is simple2code.com
Entered String is: This is simple2code.com
Notice that to get the string from the user, we use getline()
function that takes two arguments – input stream (cin) and location of the line to be stored (str).
C++ String Functions
There are different functions provided by the string class to manipulate strings in a program. The cstring class defines these functions and we need to include cstring header file in a program. Let us see some of these functions with examples.
Function | Description |
---|---|
strcpy(str1, str2); | It copies string2 content in string1. |
strcmp(str1, str2); | It is a comparison function. returns 0, if str1 = str2 returns less than 0, if str1<str2 returns greater than 0, if str1>str2 |
strcat(str1, str2); | It joins str2 into the end of str1. |
strlen(str); | It returns the length of the string passed. |
Example of the above string function in C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | #include <iostream> #include <cstring> using namespace std; int main() { char str1[10] = "Mark"; char str2[10] = "John"; char str3[10]; int len; //copy string2 to string3 strcpy(str3, str2); cout << "strcpy( str3, str2) : " << str3 << endl; //concatinate string1 and string2 strcat(str1, str2); cout << "strcat( str1, str2): " << str1 << endl; //length of a string1 len = strlen(str1); cout << "strlen(str1) : " << len << endl; //compare: returns 0 since str2 = str3 cout << "strcmp(str2, str3) : " << strcmp(str2, str3) << endl; return 0; } |
Output:
strcpy( str3, str2) : John
strcat( str1, str2): MarkJohn
strlen(str1) : 8
strcmp(str2, str3) : 0
Passing String to a Function
We pass the string in a similar way like we pass an array to a function. We will see an example where we will learn to pass two different strings in a function.
It is a basic example, where we create two print functions and take the user input for two different strings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | #include <iostream> using namespace std; //function prototype void print(string); void print(char *); int main() { string str1; char str2[100]; cout << "Enter First string: "; getline(cin, str1); cout << "Enter Second string: "; cin.get(str2, 100); print(str1); print(str2); return 0; } void print(string str) { cout << "\nString is: " << str << endl; } void print(char str[]) { cout << "Char array is: " << str << endl; } |
Enter First string: This is a website
Enter Second string: It is simple2code.com
String is: This is a website
Char array is: It is simple2code.com