In this tutorial, we will learn and write a C++ program to check whether a given matrix is an identity matrix or not. To understand the coding, you should have knowledge of the following topics in C++ programming:
Identity Matrix:
A square matrix is said to be an identity matrix if each entry on its diagonal is equal to 1 and the rest are 0. Example:
Matrix of dimension 3 by 3:1 0 0
0 1 0
0 0 1
Explanation: The program takes user input for the number of rows and columns of a matrix. The values are of each element are also taken from the user.
Before entering the elements of the matrix, the program also checks for the matrix to be a square matrix. If it is a square matrix then the execution control moves to the next line but if not then the program is exited.
The program checks the diagonals matrix for 1 and the rest for 0. If the conditions are found to be true then the entered matrix is identity matrix else not.
Now let us go through a program for Identity Matrix in C++.
C++ Program to Find if an Array is an Identity Matrix
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | #include <iostream> using namespace std; int main() { int i, j, row, col, arr[10][10], flag = 0; cout << "Enter the number of rows: "; cin >> row; cout << "Enter the number of column: "; cin >> col; //Checking for square matrix if (row != col) { cout << "\nMatrix is not a square matrix. Try Again!"; exit(0); } cout << "\nEnter the elements of matrix:\n"; for (i = 0; i < row; i++) for (j = 0; j < col; j++) cin >> arr[i][j]; //Checking for diagonals and rest for (i = 0; i < row; i++) { for (j = 0; j < col; j++) { if ((arr[i][j] != 1) && (arr[j][i] != 0)) { flag = 1; break; } } } //Display accordingly if (flag == 0) cout << "\nThe entered matirix is an identity matrix.\n"; else cout << "\nThe entered matirix is NOT an identity matrix."; //Display the matrix for (i = 0; i < row; i++) { for (j = 0; j < col; j++) cout << arr[i][j] << " "; cout << "\n"; } return 0; } |
Output:
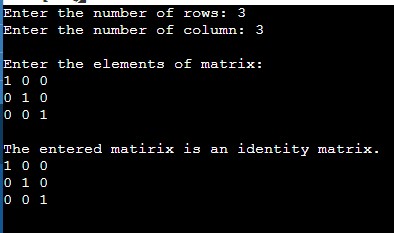