A situation may arise during coding where you need to repeat the block of code for some number of times then the loops present in C++ will come in play.
Loops allow us to execute a block of code or statement or group of statements as many times according to the user’s need. The condition is checked every time the loop is repeated. It is done by evaluating a given condition for true and false.
If true, the loop statement are repeated, if false then the loop is skipped.
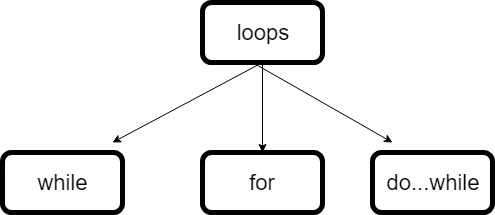
Two Types of Loops in C Programming.
1. Entry controlled loop:
In entry control loops, the conditional is checked first and then the block of code is executed. It is also called a pre-checking loop.
There are two types of entry control loop:
- for loop.
- while loop.
2. Exit controlled loop:
In exit control loop the block of code is executed first and then the condition is checked. It is also called a post-checking loop.
There is only one type loop in the exit control loop.
- do-while loop.
While Loop in C++
A while loop is a straightforward loop and is also an entry-control loop. It evaluates the condition before executing the block of the loop. If the condition is found to be true, only then the body of the loop is executed. The loop continues until the condition stated found to be false.
While loops are used when we do not know the exact number of times the iteration required beforehand. By default the while loop condition is true.
The syntax for while loop
in C++:
1 2 3 4 | while(condition) { //block of code to be executed } |
You should use a while loop, where the exact number of iterations is not known but the loop termination condition, is known.
For example and flowchart of while loop
, click here
do…while loop in C++
Sometimes it is necessary that the block or statement must be executed at least once but if the condition is initially at a false state then the block will not be executed. So for the situation where a block of code must be executed at least once a do-while loop comes into play.
It is the same as the while loop where the loop is terminated on the basis of the test condition. The main difference is that the do-while loop checks the condition at the end of the loop which allows the do-while to execute the loop at least once.
The syntax for do-while loop
in C++:
1 2 3 4 | do { //statements.. }while (condition); |
You should use do while loop if the code needs to be executed at least once.
For example and flowchart of do-while loop
, click here
for Loop in C++
The for loop has a more efficient loop structure and is an entry-control loop. The loop allows the programmer to write the execution steps together in a single line and also the specific number of times the execution is to be iterated.
It has three computing steps as shown in the syntax below.
- initialization: The first step is the initialization of the variable and is executed only once. And need to end with a semicolon(
;
). - condition: Second is condition check, it checks for a boolean expression. If true then enter the block and if false exit the loop. And need to end with a semicolon(
;
). - Increment or Decrement: The third one is the increment or decrement of the variable for the next iteration. Here, we do not need to use the semicolon at the end.
The syntax for for loop
in C++:
1 2 3 4 | for(initialization; condition; Increment or Decrement) { // Statements } |
You should use for a loop when the number of iterations is known beforehand, i.e. when the number of times the loop body is needed to be executed is known.
For example and flowchart of for loop
, click here
Loop Control Statement in C#
C# break Statement: break statement in programming is used to come out of the loop or switch statements. It is a jump statement that breaks the control flow of the program and transfers the execution control after the loop.
Click here to learn in detail
C# continue Statement: It is a loop control statement. It is used to continue the loop in the program. The use of continue inside a loop skips the remaining code and transfer the current flow of the execution to the beginning o the loop.
Click here to learn in detail
C# goto Statement: The goto statement is a jump statement that allows the user in the program to jump the execution control to the other part of the program.
Click here to learn in detail.
The Infinite Loop
As the name suggests, the infinite loop is a forever executing loop. The infinite loop repeats indefinitely and the condition never becomes false. It is also known as an indefinite loop or an endless loop.
We can use one of the Loops in C++ to turn it into infinite loop.
infinite ‘for’ loop
1 2 3 4 | for(; ;) { //body of the loop } |
infinite while loop
1 2 3 4 | while(1) { //body of the loop } |
infinite do-while loop
1 2 3 4 | do { //body of the loop }while(1); |
Example: of infinite for loop in C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | #include <iostream> using namespace std; int main () { int i; //infinite for loop for ( ; ; ) { cout << "This loop will run forever.\n"; } /*//infinite while loop while (true) { cout << "This loop will run forever.\n"; } */ return 0; } |
The compiler assume the condition to be true if the condition is absent in the loop.