In this article, we will learn how to Reverse a String in C. Before that you may go through the following topic in C.
We will look into two different Programs.
1. First one with the help of a Library function
2. Second, without the function.
Question:
How to write a program in C to Reverse a String with and without the use of library function.
1. String Reversal in C using Library function
In this c program for string reversal, we have used strrev() function to reverse entered string
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <stdio.h> #include <string.h> #define MAX_SIZE 100 int main() { char str[MAX_SIZE]; printf("Enter a string: \n"); fgets(str, MAX_SIZE, stdin); strrev(str); printf("Reverse of entered String: %s\n", str); return 0; } |
The output of reverse the string in C.
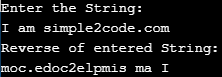
2. String Reversal in C without Library function
In this c program for string reversal, we have used while to reverse each character from the entered String.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | #include <stdio.h> #include <string.h> #define MAX_SIZE 100 int main() { char str[MAX_SIZE]; int i, j = 0, temp; printf("Enter the String:\n"); fgets(str, MAX_SIZE, stdin); i = 0; j = strlen(str) - 1; while (i < j) { temp = str[i]; str[i] = str[j]; str[j] = temp; i++; j--; } printf("Reverse of entered String: %s\n", str); return 0; } |
The output of reversing the string in C Programming.
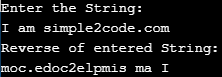