This tutorial contains about the String in C programming. You will learn about the declaration, initialization of String, and the use of string with examples.
What is String?
A string is defined as a series of characters or an array of characters(terminated by a null character ‘\0’). Each character present in the array occupies one byte of memory. The manipulation of text(words or sentences) in a c program is done by String.
The termination character that is the ‘\0’ character is essential to add in the string as it is the only way to specify where the particular string ends.
Declaring and Initializing a String in C
You can declare the string in two ways:
1. By character array: Here we declare the character array with a single character as one element within the single quote. Since the last element of the array needs to be a null character so the size of the array is one more than the number of characters used. Hence always take one size more than you actually need in an array for the null character.
1 2 | char str[8] = {'p','r','o','g','r','a','m','\0'}; char str[] = {'p','r','o','g','r','a','m','\0'}; |
Memory presentation of the above character array in C/C++:
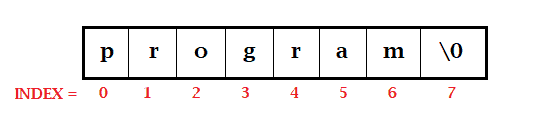
2. By String Literal: In this case, we can simply write the complete string(words or sentence) at once that is by the string literal. Here, you don’t have to add a null character at the end, the compiler will append ‘\0’ at the end of the string.
1 2 | char str[]="program"; char str[15]="simple2code"; |
In both cases, you can either give the array size or you can just leave it blank and it will take the size according to the number of characters you will initialize it with.
For character %s
format specifier is used in a program.
Let us see a simple example:
String program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | #include <stdio.h> int main () { //By character array char arr[13] = {'S','i','m','p','l','e','T','o','C','o','d','e','\0'}; printf("This Website: %s\n", arr); //By String Literal char str[] = "Simple2code Tutorial"; printf("This Website Tutorial: %s\n", str); return 0; } |
Output:
This Website: SimpleToCode
This Website Tutorial: Simple2code Tutorial
How to Read String from the User.
Taking input from the user is done by a built-in library function scanf()
. Scanf() keeps on taking the input from the user until it encounters whitespace (space, newline, tab, etc.).
NOTE that we do not use the & operator(use for address) in scanf()
function, since it is an array of characters and the name (str) itself indicates the base address of the array (string).
String C Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> int main() { char str[20]; //taking input printf("Enter Stream: "); scanf("%s", str); //displaying the input printf("Stream is: %s", str); return 0; } |
Output:
Enter Stream: Computer Science
Stream is: Computer
In the above program, only the Computer is displayed when we try to print the string that is because of the white space in between. Now this could be overcome by changing a line of code that is you need to write scanf("%[^\n]s", str)
instead of scanf("%s", str)
. It will allow you to store the string until a newline(\n) is encountered.
Let see it in a program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> int main() { char str[20]; //taking input printf("Enter Stream: "); scanf("%[^\n]s", str);//replaced code //displaying the input printf("Stream is: %s", str); return 0; } |
Output:
Enter Stream: Computer Science.
Stream is: Computer Science.
Passing String to a Function
Passing a string to a function is done in the same way as passing an array to a function.
Example: C program to pass a string to a function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | #include <stdio.h> void displayFunc(char str[]) { printf("Stream is: %s", str); } int main() { char str[20]; //taking input printf("Enter Stream: "); scanf("%[^\n]s", str); //calling function displayFunc(str); return 0; } |
Output:
Enter Stream: Computer Science.
Stream is: Computer Science.
String with Pointers
We have already seen the use of pointers with arrays. In the same way, we can use a pointer with a string in C to point the String. We can manipulate the elements of a string with the pointer.
Example 1: String and Pointer
1 2 3 4 5 6 7 8 9 10 11 12 | #include<stdio.h> int main () { char str[11] = "Simple2Code"; //ptr pointer points to str String char *ptr = str; printf("String present in str: %s",ptr);//str string is printed return 0; } |
Output:
String present in str: Simple2Code
Example 2: String and Pointer
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | #include <stdio.h> int main(void) { char str[] = "Simple Code"; printf("Oth position: %c\n", *str); // S printf("3th position: %c\n", *(str+3)); // p char *strPtr; strPtr = str; printf("Oth position: %c\n", *strPtr); // S printf("7th position: %c", *(strPtr+7)); // C } |
Output:
Oth position: S
3th position: p
Oth position: S
7th position: C
As you can see we can manipulate the element with the help of the pointer too. We can increment or decrement the pointer in order to get to the required positioned element.