In this tutorial, you will learn how to count the number of Digits in an integer in C programming. You will learn various approaches possible to Count the Number of Digits. But before that, you need to have knowledge of the following topics in C programming.
Explanation:
The following programs simply take the user input and display how many digits are present in that integer.
Example: Consider an Integer “256“, The number of digits present here is 3. Therefore the program displays 3 as a result of the program.
1. C Program to Count the Number of Digits using while loop
The same method is applied if you want to do it with the for a loop. Instead of while loop, use for loop in the calculation part, the rest remains the same
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include <stdio.h> int main() { // variable declaration int num; int count = 0; //user Input printf("Enter the integer: "); scanf("%d", &num); //calculation while (num != 0) { num = num / 10; count++; } printf("The number of digits present are: %d", count); return 0; } |
Output:
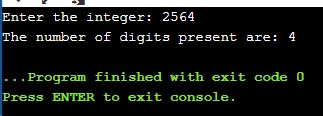
2. C Program to Count the Number of Digits using math library function
Here, we will not use any loops to calculate the result. We will use one of the inbuilt functions provided by the math library in C language and in a single line. The logarithmic function will be helpful in such a program.
log10(num)+1, where log10() is the predefined function in math.h header file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | #include <stdio.h> #include <math.h> int main() { // variable declaration int num; int count = 0; //user Input printf("Enter the integer: "); scanf("%d", &num); //calculation count = (num == 0) ? 1 : log10(num) + 1; printf("The number of digits present are: %d", count); return 0; } |
Output:
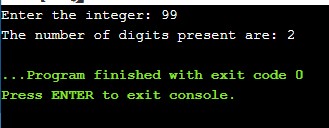
3. C Program to Count the Number of Digits using function
A separate function is created in the program and the integer value is passed as an argument in the program as shown below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | #include <stdio.h> int calculate(int n); //function prototype int main() { // variable declaration int num; int result = 0; printf("Enter the integer: "); scanf("%d", &num); result = calculate(num); printf("The number of digits present are: %d", result); return 0; } int calculate(int n) { int count = 0; while (n != 0) { n = n / 10; count++; } return count; } |
Output:
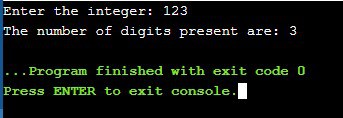
4. C Program to Count the Number of Digits using recursion
Here static is used to count. As the recursion function calls itself again and again until the condition is full-filled, so static variable count is declared so that its value doesn’t initialize again and again after the first call.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | #include <stdio.h> int calculate(int n); //function prototype int main() { // variable declaration int num; int result = 0; printf("Enter the integer: "); scanf("%d", &num); result = calculate(num); printf("The number of digits present are: %d", result); return 0; } int calculate(int n) { static int count = 0; if (n > 0) { count = count + 1; return calculate(n / 10); } else { return count; } } |
Output:
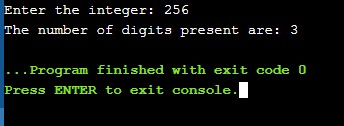