Recursion refers to the process when a function calls itself inside that function directly or indirectly or in a cycle. And such functions are called recursive functions. However, the crucial part is the termination condition that is given to the recursive function because it might go into an infinite loop.
If the termination is not processed properly then that will lead to stack overflow (recursion invokes stack memory) which will lead to a system crash. It could be a little hard to understand the recursive code but the code becomes shorter than the iterative code.
1 2 3 4 5 6 7 8 9 10 11 12 13 | void recursion() { ...... recursion(); //calling itself ...... } int main() { ...... recursion(); ...... } |
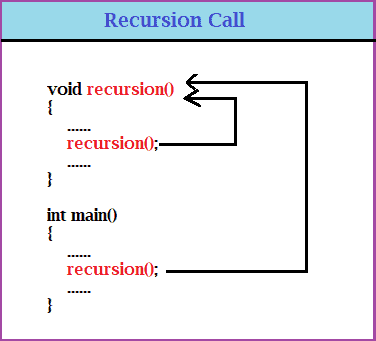
Recursion is very useful in performing mathematical calculations such as during the calculation of factorial of a number, Fibonacci series problem, etc. Although any problem that can be performed by recursion can also be done iteratively.
But sometimes it is best-suited to use recursion such as the tower of Hanoi, Factorial and Fibonacci calculation, etc.
What is base condition?
In recursion, the larger problem is solved in terms of smaller problems, and the larger value is solved by converting into a smaller value still the base condition is reached by the function. It is the condition when the computing is complete and needs to get out of the function.
1 2 3 4 5 6 7 8 | int factorial_Number(int n) { if (n == 1) //base condition return 1; else return (n* factorial_Number(n - 1)); } |
What do you mean by direct and indirect recursion?
When the function call itself (same function), it is called a direct call. When some function is called inside another function then it is said to be an indirect call. Relate to the example given below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | // Direct recursion void direct_Func() { .... .. . direct_Fun(); ... .. . } // Indirect recursion void indirect_Func1() { .... .. . indirect_Func2(); .... .. . } void indirect_Func2() { .... .. . indirect_Func1(); .... .. . } |
Factorial Program in C using Recursion
factorial of n (n!) = n * (n-1) * (n-2) * (n-3)….1
factorial of 5 (n!) = 5 * 4 * 3 * 2 * 1
NOTE: Factorial of 0 (0!) = 1
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | /*Find Factorial of a Number using Recursion*/ #include <stdio.h> int factorial_Number(int); int main() { int num, result; //taking user input printf("Enter the Number to Find the Factorial of: "); scanf("%d", &num); //Calling the user defined function result = factorial_Number(num); //Displaying factorial of input number printf("Factorial of %d is: %d", num, result); return 0; } //Function int factorial_Number(int n) { //condition to exit if (n == 1) return 1; else return (n* factorial_Number(n - 1)); //recurion: calling function itself } |
Output:
Enter the Number to Find the Factorial of: 5
Factorial of 5 is: 120