In this tutorial, we will write a program to convert binary to decimal in C. Before that, you must have knowledge of the following topics in C.
Binary number
The binary numbers are based on 0 and 1, so it is a base 2 number. They are the combination of 0 and 1. For example, 1001, 110101, etc.
Decimal Number
These are the numbers with a base of 10, which ranges from 0 to 9. These numbers are formed by the combination of 0 to 9 digits such as 24, 345, etc.
Here is the chart where for the equivalent value of decimal and binary numbers.
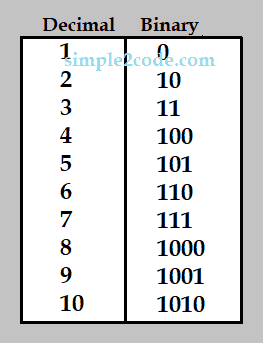
Example:
Input: 101
Output: 5
Input: 111
Output: 7
Now let us go through a program for the conversion of binary to decimal in C using while loop.
C Program to Convert Binary to Decimal using while loop
In this program a math function pow()
is used, it gives the power calculation and a header file math.h
is required to add the beginning of the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | #include <stdio.h> #include <conio.h> #include <math.h> int main() { int binaryNum, deciNum = 0, i = 0, rem; printf("Enter a binary number: "); scanf("%d", &binaryNum); while (binaryNum != 0) { rem = binaryNum % 10; deciNum = deciNum + rem* pow(2, i); i++; binaryNum = binaryNum / 10; } printf("Equivalent Decimal Value: %d", deciNum); getch(); return 0; } |
Output:
Enter a binary number: 1111
Equivalent Decimal Value: 15
Convert Binary to Decimal in C without pow() Function
Although if you do not want to use the pow() function then you simply replace the while loop of the above program with the following while loop code and others remain the same.
1 2 3 4 5 6 7 | while (binnum != 0) { rem = binnum % 10; decnum = decnum + (rem *i); i = i * 2; binnum = binnum / 10; } |
It will produce the same output as the above. Also, you do not need to include math.h
header file.