In this tutorial, we will write a program to print ASCII value of a character in C programming. Before that, you should have knowledge on the following topic in C.
ASCII stands for American Standard Code for Information Interchange. It is a 7-bit character set that contains 128 (0 to 127) characters. It represents the numerical value of a character.
Example: ASCII value for character A is 65, B is 66 and a is 97, b is 98, and so on.
C Program to Print ASCII Value of a Character
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> #include <conio.h> int main() { char ch; printf("Enter a Character: "); scanf("%c", &ch); printf("Its ASCII Value: %d", ch); getch(); return 0; } |
Output:
Enter a Character: A
Its ASCII Value: 65
C Program to print ASCII value of all Characters
Source Code: Print the ASCII value of all the characters in C.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> #include <conio.h> int main() { int i; printf("ASCII Value of all the Characters.\n"); for (i = 0; i < 255; i++) printf("ASCII value of %c: %i\n", i, i); getch(); return 0; } |
Output: You will get the list of all the characters starting from 0 to 255 on the output display screen.
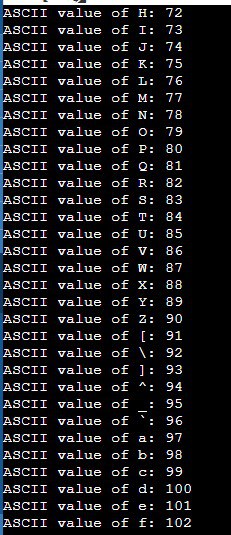