In this tutorial, we will write a java program to display Floyd’s Triangle. Before that, you should have knowledge on the following topic in Java.
Java Program to Print Floyd’s Triangle
The program asks the user for the number of rows for the Floyd triangle. Using inner and outer for loop it prints the pattern in java.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | //Print Floyd's Triangle in java import java.util.Scanner; public class Main { public static void main(String args[]) { int range, i, j, k = 1; Scanner scan = new Scanner(System.in); System.out.print("Enter the no. of rows: "); range = scan.nextInt(); System.out.print("Floyd's Triangle:\n"); for (i = 1; i <= range; i++) { for (j = 1; j <= i; j++, k++) { System.out.print(k + " "); } System.out.println(); } } } |
Output:
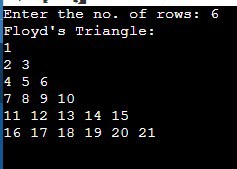