In this tutorial, we will write a C program to convert decimal to binary. Before that, you must have knowledge of the following topics in C.
Binary number
The binary numbers are based on 0 and 1, so it is a base 2 number. They are the combination of 0 and 1. For example, 1001, 110101, etc.
Decimal Number
These are the numbers with a base of 10, which ranges from 0 to 9. These numbers are formed by the combination of 0 to 9 digits such as 24, 345, etc.
Here is the chart where for the equivalent value of decimal and binary numbers.
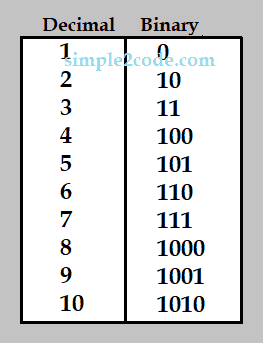
Example:
Input: 5
Output: 101
Input: 15
Output: 1111
Now let us go through a program for the conversion of decimal to binary in C using while loop.
C Program to Convert Decimal to Binary using While loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | #include <stdio.h> #include <conio.h> int main() { int deciNum, binaryNum[30], i = 0; printf("Enter a decimal number: "); scanf("%d", &deciNum); while (deciNum != 0) { binaryNum[i] = deciNum % 2; i++; deciNum = deciNum / 2; } printf("Equivalent Binary Value: "); for (i = (i - 1); i >= 0; i--) printf("%d", binaryNum[i]); getch(); return 0; } |
Output:
Enter a decimal number: 13
Equivalent Binary Number: 1101
C Program to Convert Decimal to Binary without using Array
Source code: Decimal to Binary without using Array in C.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | #include <stdio.h> #include <conio.h> int main() { int deciNum, binaryNum = 0, mul = 1, rem; printf("Enter any decimal number: "); scanf("%d", &deciNum); while (deciNum > 0) { rem = deciNum % 2; binaryNum = binaryNum + (rem *mul); mul = mul * 10; deciNum = deciNum / 2; } printf("Equivalent Binary Value: %d", binaryNum); getch(); return 0; } |
After the successful execution of the above program, it will produce the same output as the above program.