What is Multithreading?
The execution of multiple threads or tasks is called multithreading. The main purpose of multithreading is to achieve the simultaneous execution of more than two tasks so to maximize the use of CPU time.
Thread is a light-weight sub-process or we can say that it is the smallest part of the process. All threads in a program have different execution paths carrying their individual tasks. This is one of the advantages of threads that threads are independent carrying their respective tasks without affecting the others.
Thread Life Cycle:
The life cycle of a thread means the various stages a thread goes during its lifetime.
According to the sun, there are four states. They are: new, runnable, non-runnable, and terminated.
For better understanding, let us consider the running state also.
- New
- Runnable
- Running
- Non-Runnable (Blocked)
- Terminated
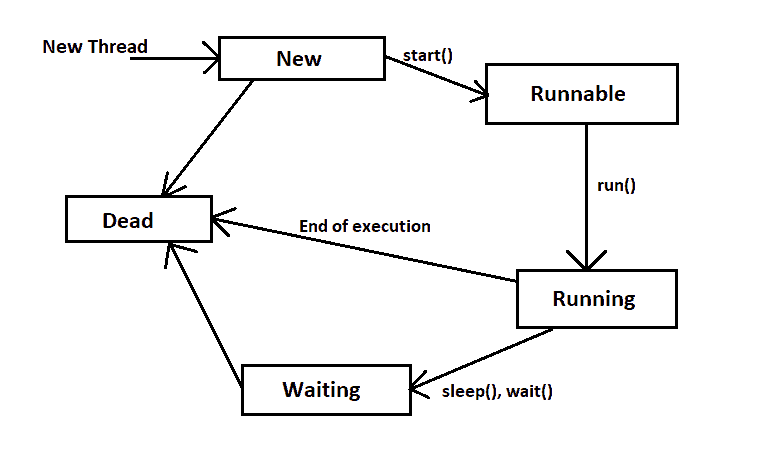
- New:
It is referred to as born thread that is it is in its new state. It won’t do anything until the start() method is invoked. - Runnable:
After the start() method is invoked, the thread will be in its runnable state. But the control is under the scheduler to finish the execution. - Running:
The thread is considered in its running state when it starts executing. The scheduler selects the thread and executes it in the application. - Non-runnable:
In this state, the thread has to wait. When the multiple threads run in an application, one thread has to wait until the other thread gets executed. - Terminated:
After the completion of the process of thread, it is in its dead state or we can say the thread is terminated.
Thread priorities:
In Java thread priorities determines which thread is to execute first and it depends in their priorities.
Context Switching is switching from one state to another.
The higher priority threads are considered to be more important in the application and the operating system executes it first. The range of priorities in java:
- MIN_PRIORITY (a constant of 1)
- MAX_PRIORITY (a constant of 10).
- And default priorities: NORM_PRIORITY (a constant of 5).
Creating a thread:
There are two ways to create a thread:
- By extending Thread class.
- By implementing the Runnable interface.
Some of the commonly used Thread class;
public start(): start a thread by calling its run() method.
public run(): Entry point for the thread.
public isAlive(): Determine if a thread is still running.
public join(): Wait for a thread to terminate.
public getName(): It is used for Obtaining a thread’s name.
public getPriority(): Obtain a thread’s priority.
public sleep(): suspend a thread for a period of time.
public int getId(): returns the id of the thread.
public void stop(): is used to stop the thread(deprecated).
1. By extending Thread class.
The thread class provides methods and also constructors for creating and performing some operation on a thread. In this process, we create a class and extend java.lang.Thread class.
- First, we override the run() method that is present in the thread class and then put the code inside it.
- Secondly, we create an object for our class and call the start() method for the execution of a thread.
Syntax for Thread class:
1 2 | run(): public void run( ); start(): void start( ); |
Java program of Thread class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | class MultiThread extends Thread //extends thread { public void run() { System.out.println("Successful."); } } public class Main { public static void main(String args[]) { MultiThread th = new MultiThread(); th.start(); } } |
Output:
1 | Successful. |
2. By implementing the Runnable interface:
If one of the class’ instance is intended to be executed by a thread then this can be achieved by implementing a Runnable interface. Implementation of java.lang. Runnable interface has one method called run().
- Here the first step is to provide a program with a run() method that is to override this method and put the block of code or the logic inside it.
- Secondly, the Thread object is instantiated and once the thread object is created, we can call the start() method to start the thread which will execute run() method.
Syntax for Runnable interface:
1 2 | run(): public void run( ); start(): void start( ); |
Java program of Runnable interface:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | class MultiThread implements Runnable { public void run() { System.out.println("Successful"); } } public class Main { public static void main(String args[]) { MultiThread mth=new MultiThread(); Thread th =new Thread(mth); th.start(); /*or we can also write this above code as Thread th = new Thread(new MultiThread()); th.start(); */ } } |
Output:
1 | Successful |