It is one of the main features of Object-Oriented Programming (OOP). Inheritance is one of the processes or mechanisms in OOP in which one class(sub-class) acquires the properties(data members) and functionalities(methods) of another class(parent-class).
Importance of Inheritance:
- For Code Reusability.
- For Method Overriding (to achieve runtime polymorphism).
Important terms:
- Super Class: The class whose properties and functionalities are inherited by the Subclass is known as superclass(a parent class or a base class).
- Sub Class: The class that inherits the property and behavior of the other class(Superclass) is known as subclass( a derived class, extended class, or child class). The subclass can have its own fields and methods along with the fields and methods of the superclass.
- Reusability: Inheritance supports the concept of “reusability”. This feature allows us to use the methods in the sub-class that is already present in the superclass, saving the time to rewrite the code.
- extends: extends is the keyword that’s used by the base class so that it can inherit the properties of the superclass.
Syntax:
1 2 3 4 5 6 7 8 | class Super { //field and methods } class sub extends Super { //its own field and methods } |
Basic Example for Inheritance:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | class Dog { void dogName() { System.out.println("My Name is Bruno"); } } class Breed extends Dog { void myBreed() { System.out.println("German Shepard"); } } public class Main { public static void main(String args[]) { Breed d = new Breed(); d.dogName(); d.myBreed(); } } |
Output:
My Name is Bruno
German Shepard
In the above example, the Breed class inherits the method ‘dogName()‘ from the superclass with the help of a keyword ‘extends’.
Note: The Object is created for only the Breed class but not for the Dog class. That is we can access the methods without creating the object of the superclass.
Although there are some concepts you need to know before inheriting the superclass, the method that the user wishes to inherit from the superclass cannot be private and also the superclass itself should not be declared private otherwise it cannot be inherited. ‘protected’ methods can be inherited.
Types of Inheritance:
There are three types of inheritance in java on the basis of class. They are :
- single inheritance
- multi level inheritance
- hierarchical inheritance
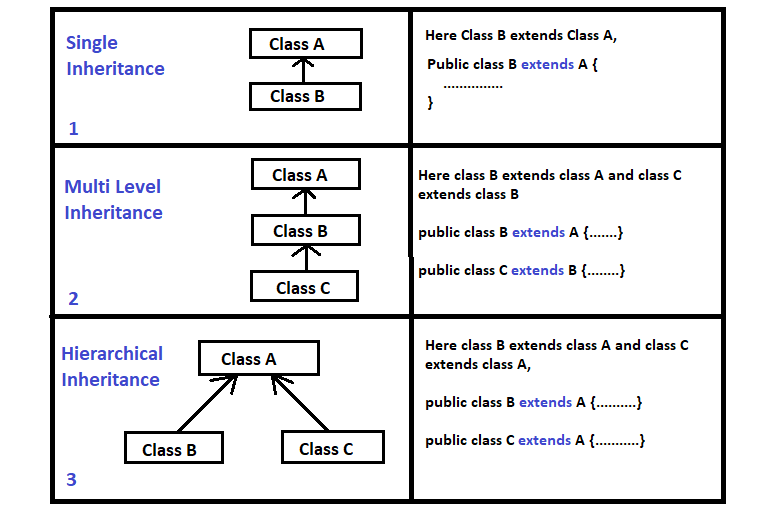
Java does not support Multiple and Hybrid Inheritance but can be achieved by Interfaces.
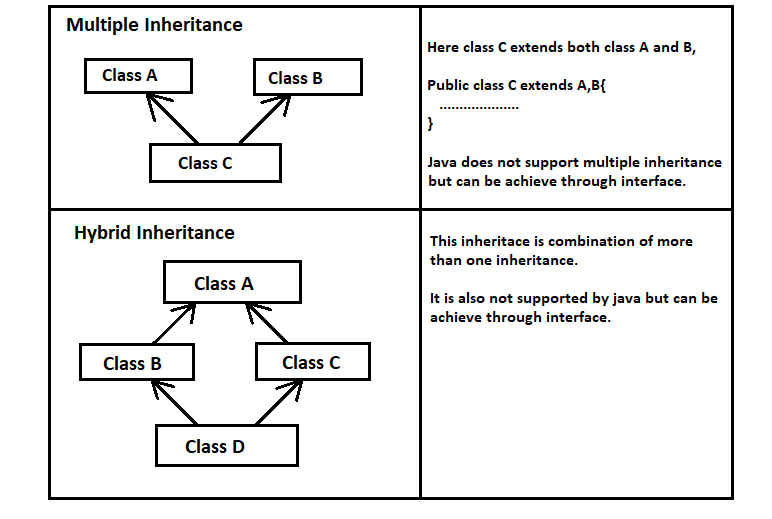
1. Single Inheritance:
It refers to an inheritance where a child inherits or extends a single-parent class.
Here class B extends class A.
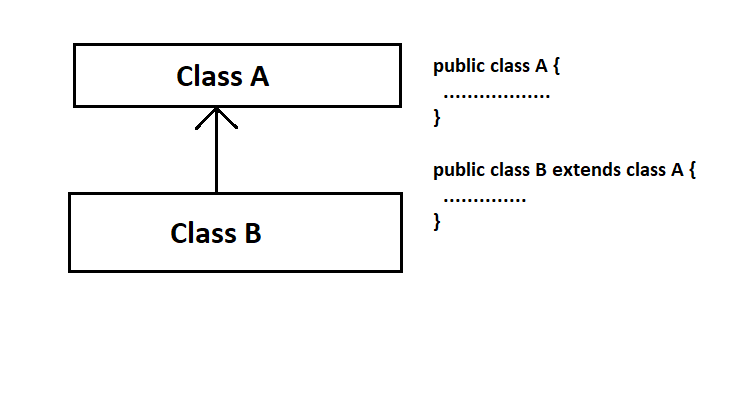
2. Multilevel inheritance:
It refers to an inheritance where one class inherits from the derived class and making that derived class the superclass for the new class.
Here class C inherits from class B and class B inherits from class A.
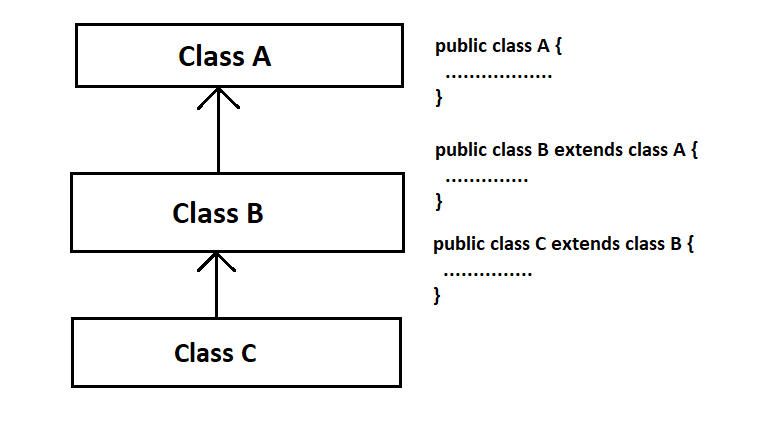
3. Hierarchical Inheritance:
It refers to a relationship where more than one class can inherit or extends the same class.
Here class B and class C inherit class A.
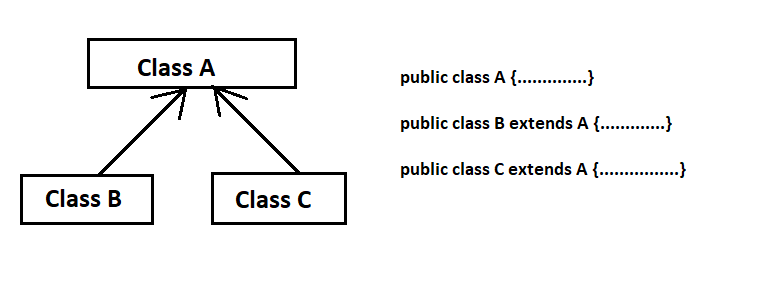
4. Multiple Inheritance:
In this relationship, one class(derived class) can inherit from more than one superclass. Java doesn’t support multiple inheritance.
check the diagram above.
5. Hybrid Inheritance:
A combination of more than one type of inheritance in a program is called Hybrid Inheritance. It is also not supported by Java.
check the diagram above.
Click here to learn about each of the inheritances with examples.
Constructors and super Keyword in Inheritance.
In Inheritance, a subclass inherits all the members that are its fields, methods, and nested classes from its superclass. But Constructors are not members, so they cannot be inherited by subclasses, but the constructor of the superclass can be invoked from the subclass with the help of a super keyword.
super Keyword refers directly to the superclass in its hierarchy. It is similar to this Keyword.
super is used to invoke the superclass constructor from a subclass. And also used when the members having the same name in the superclass and subclass and therefore need to differentiate between them.
Syntax of super Keyword:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | class SuperclassTest { int num1, num2; SuperclassTest(int num1, int num2) { this.num1 = num1; this.num2 = num2; } public void getNum() { System.out.println("Numbers in super class are: " + num1 + " and: " + num2); } } public class Subclass extends SuperclassTest { Subclass(int num1, int num2) { super(num1, num2); //use of super } public static void main(String argd[]) { Subclass s = new Subclass(24, 30); s.getNum(); } } |
Output:
Numbers in super class are: 24 and: 30
Java Program for Super keyword
Example of a super keyword to differentiate the members of the superclass from a subclass.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | class SuperClass { int id = 20; public void display() { System.out.println("Super Class display method"); } } public class SubClass extends SuperClass { int id = 10; public void display() { System.out.println("Sub Class display method"); } public void myMethods() { // Instantiating subclass SubClass s = new SubClass(); // display method and value of sub class s.display(); System.out.println("Sub Class id:" + s.id); // display method and value of super class using super super.display(); System.out.println("Super Class id::" + super.id); } public static void main(String args[]) { SubClass sub = new SubClass(); sub.myMethods(); } } |
Output:
Base Class display method
Sub Class id:10
Super Class display method
Super Class id::20