The access modifiers in Java specifies the accessibility or visibility or scope of a field, method, constructor, or class. Java defines four access modifiers:
Following shows their accessibility,
- default (Same class, Same package)
- private (Same class)
- protected ( Same class, Same package, Subclasses)
- public ( Same class, Same package, Subclasses, Everyone)
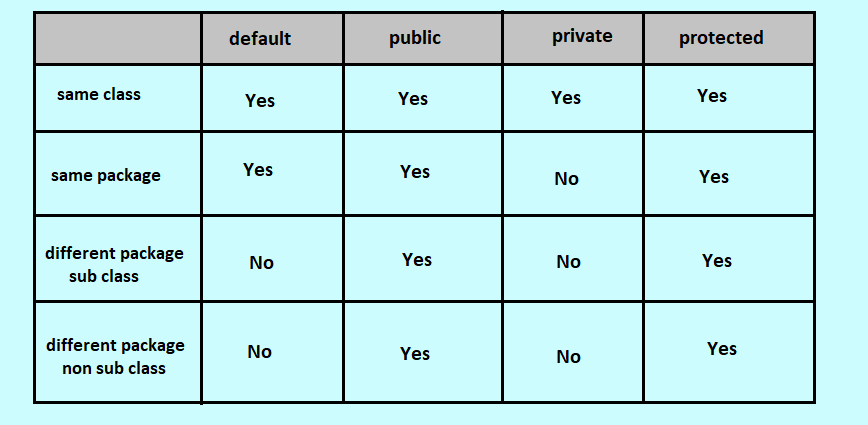
1. default:
Default access modifiers mean, no modifiers are specified to the class, method, or data members. These parts where no access modifiers are specified that are having a default modifier are only accessible within the same package.
let us see an example:
In this example we created two packages ‘name’ and ‘mainPack’. ‘name’ package contains the default access modifiers class and method. Now if we import this package in the mainPack then we will get a compile-time error because the class ‘Names’ is only accessible within that package.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | //save by Names.java package name; class Names { void myName() { System.out.println("I am John"); } } //save by Main.java package mainPack; import pack.*; class Main { public static void main(String args[]) { Names n = new Names(); n.myName();//Compilation Error } } |
2. private:
It is specified using the private keyword. Variables, Methods, and constructors that are declared private modifiers are only accessed within the declared class itself. Any other package cannot access them. Classes and Interfaces cannot be declared as private but can only be applied to inner classes.
In this example, a class named ‘Name’ contains a private method called ‘display()’ which cannot be accessed in another class Main, showing the error result.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | class Name { private void display() { System.out.println("I am John"); } } public class Main { public static void main(String args[]) { Name n = new Name(); //accesing the private method of another class n.display(); } } |
Output:
1 2 | java:15: error: display() has private access in Name n.display(); |
3. protected:
Variables, methods, and constructors, which are declared protected using the keyword ‘protected‘.
Protected data methods and members are only accessible by the classes of the same package and the subclasses present in any package. Classes and Interfaces cannot be declared protected.
Let us see it in an example:
The two packages Pack1 and pack2 are declared where pack1 contains the protected method ‘display()’ and is accessed in pack2 by importing pack1 in it. In this example, the protected method ‘display()’ declared in a class ‘Name’ is accessed by class Main.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | //save by Name.java package pack1; public class Name { protected void display() { System.out.println("I am John"); } } //save by Main.java package pack2; import pack1.*; //importing from pack1 //inheriting Name class class Main extends Name { public static void main(String args[]) { Name obj = new Name(); obj.display(); } } |
Output:
I am John
Note:
Methods and fields cannot be declared protected in an Interface.
4. Public:
Declaring fields and methods or classes public means making it accessible everywhere in a program or in an application. A public entity can be accessed from class in a program. However, if the needs to access the public class from the different packages then in order to access it that package must be imported first.
Let us see it in an example:
Two classes Mult and Main are created for different packages, named pack1 and pack2. Mult class is declared public and its method ‘multTwoNumber’ is also declared public and so to access it in a ‘Main’ class its package must be imported that is pack1 is imported in Main.java.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | //saved by Mult.java package pack1; public class Mult { public int multTwoNumber(int x, int y) { return x*y; } } //saved by Main.java package pack2; import pack1.*; class Main { public static void main(String args[]) { Mult mul = new Mult(); System.out.println(mul.multTwoNumber(5, 10)); } } |
Output:
50