This post focuses on the various Java pattern program specifically Top 10 Different Star Pattern Programs in Java. Patterns are one of the easiest ways to improve the coding skills for java. The frequent use of loops can increase your skills and printing pattern in order. This article covers various star patterns. Other are:
Let’s begin:
Printing Star Patterns in Java
Pattern programs in java: Pattern 1
1 2 3 4 5 6 7 | Half Pyramid: * * * * * * * * * * * * * * * * * * * * * |
Lets understand the coding.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | //Pattern half Pyramid public class Pattern1 { public static void main(String[] args) { int row = 6; System.out.println("Half Pyramid:"); for(int i = 1; i <= row; ++i) { for(int j = 1; j <= i; ++j) { System.out.print("* "); } System.out.println(); } } } |
Pattern programs in java: Pattern 2
1 2 3 4 5 6 7 | Inverted Half Pyramid: * * * * * * * * * * * * * * * * * * * * * |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | //Pattern Inverted Half Pyramid public class Pattern2 { public static void main(String[] args) { int rows = 6; System.out.println("Inverted Half Pyramid:"); for(int i = rows; i >= 1; --i) { for(int j = 1; j <= i; ++j) { System.out.print("* "); } System.out.println(); } } } |
Pattern programs in java: Pattern 3
1 2 3 4 5 6 | * *** ***** ******* ********* *********** |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | //Pattern full pyramid public class Main { public static void main(String[] args) { int rows = 6; System.out.println(); for (int i = 0; i < rows + 1; i++) { for (int j = rows; j > i; j--) { System.out.print(" "); } for (int k = 0; k < (2 * i - 1); k++) { System.out.print("*"); } System.out.println(); } } } |
Pattern programs in java: Pattern 4
1 2 3 4 5 6 | Enter the number of rows: 5 * * * * * * * * * * * * * * * |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | import java.util.Scanner; public class pattern4 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i= 0; i<= rows-1 ; i++) { for (int j=0; j<=i; j++) { System.out.print(" "); } for (int k=0; k<=rows-1-i; k++) { System.out.print("*" + " "); } System.out.println(); } sc.close(); } } |
Pattern programs in java: Pattern 5
1 2 3 4 5 6 7 8 9 10 | Enter the number of rows: 5 * * * * * * * * * * * * * * * * * * * * * * * * * |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | import java.util.Scanner; public class Pattern5 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i= 0; i<= rows-1 ; i++) { for (int j=rows-1; j>=i; j--) { System.out.print(" "); } for (int k=0; k<=i; k++) { System.out.print("*" + " "); } System.out.println(""); } for (int i= 0; i<= rows-1 ; i++) { for (int j=-1; j<=i; j++) { System.out.print(" "); } for (int k=0; k<=rows-2-i; k++) { System.out.print("*" + " "); } System.out.println(""); } sc.close(); } } |
Pattern programs in java: Pattern 6
The half diamond pattern in java.
1 2 3 4 5 6 7 8 9 10 | Enter the number of rows: 5 * * * * * * * * * * * * * * * * * * * * * * * * * |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | import java.util.Scanner; public class Pattern6 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i= 0; i<= rows-1 ; i++) { for (int j=0; j<=i; j++) { System.out.print("*"+ " "); } System.out.println(""); } for (int i=rows-1; i>=0; i--) { for(int j=0; j <= i-1;j++) { System.out.print("*"+ " "); } System.out.println(""); } sc.close(); } } |
Pattern programs in java: Pattern 7
The left half diamond pattern in java.
1 2 3 4 5 6 7 8 9 10 | Enter the number of rows: 5 * ** *** **** ***** **** *** ** * |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | import java.util.Scanner; public class Pattern7 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i= 1; i<= rows ; i++) { for (int j=i; j < rows ;j++) { System.out.print(" "); } for (int k=1; k<=i;k++) { System.out.print("*"); } System.out.println(""); } for (int i=rows; i>=1; i--) { for(int j=i; j<=rows;j++) { System.out.print(" "); } for(int k=1; k<i ;k++) { System.out.print("*"); } System.out.println(""); } sc.close(); } } |
Pattern programs in java: Pattern 8
Half hourglass pattern in java
1 2 3 4 5 6 7 8 9 10 11 | Enter the number of rows: 5 * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | import java.util.Scanner; public class Pattern8 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i= rows-1; i>= 0; i--) { for (int j=i; j>=0; j--) { System.out.print("*"+ " "); } System.out.println(""); } for (int i=0; i<= rows-1; i++) { for(int j=i; j >= 0;j--) { System.out.print("*"+ " "); } System.out.println(""); } sc.close(); } } |
Pattern programs in java: Pattern 9
Hourglass pattern in java.
1 2 3 4 5 6 7 8 9 10 11 | Enter the number of rows: 5 * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | import java.util.Scanner; public class Pattern9 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i= 0; i<= rows-1 ; i++) { for (int j=0; j <i; j++) { System.out.print(" "); } for (int k=i; k<=rows-1; k++) { System.out.print("*" + " "); } System.out.println(""); } for (int i= rows-1; i>= 0; i--) { for (int j=0; j< i ;j++) { System.out.print(" "); } for (int k=i; k<=rows-1; k++) { System.out.print("*" + " "); } System.out.println(""); } sc.close(); } } |
Pattern programs in java: Pattern 10
Hollow triangle star pattern in java.
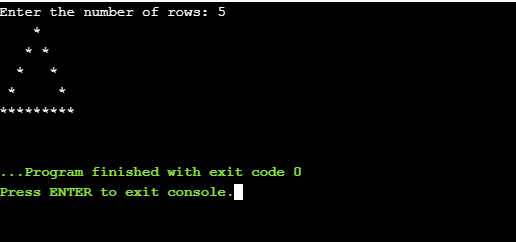
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | import java.util.Scanner; public class Pattern10 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i=1; i<= rows ; i++) { for (int j = i; j < rows ; j++) { System.out.print(" "); } for (int k = 1; k <= (2*i -1) ;k++) { if( k==1 || i == rows || k==(2*i-1)) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(""); } sc.close(); } } |