This post focuses on the various Java pattern program specifically Top 10 Different Number Pattern Programs in Java. Patterns are one of the easiest ways to improve the coding skills for java. The frequent use of loops can increase your skills and printing them in order.
This article covers various Numeric pattern programs. Other are:
Let’s begin:
Number Patterns in Java
Pattern programs in java: Pattern 1
1 2 3 4 5 6 | 1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 1 2 3 4 5 6 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | //Pattern with Number public class Patter1 { public static void main(String[] args) { int rows = 6; for(int i = 1; i <= rows; ++i) { for(int j = 1; j <= i; ++j) { System.out.print(j + " "); } System.out.println(); } } } |
Pattern programs in java: Pattern 2
1 2 3 4 5 6 7 8 9 10 | 1 2 4 3 6 9 4 8 12 16 5 10 15 20 25 6 12 18 24 30 36 7 14 21 28 35 42 49 8 16 24 32 40 48 56 64 9 18 27 36 45 54 63 72 81 10 20 30 40 50 60 70 80 90 100 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | //Pattern with Number public class Pattern2 { public static void main(String[] args) { int lines=10, i=1, j; for(i=1;i<=lines;i++) { for(j=1;j<=i;j++) { System.out.print(i*j+" "); } System.out.println(""); } } } |
Pattern programs in java: Pattern 3
1 2 3 4 5 | 12345 1234 123 12 1 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | //Pattern with Numbers public class Pattern3 { public static void main(String[] args) { int count = 5; for(int i = count; i > 0 ; i-- ) { for(int j = 1; j <= i ; j++) { System.out.print(j); } System.out.println(""); } } } |
Now with user input:
Pattern programs in java: Pattern 4
1 2 3 4 5 6 7 8 9 10 | Enter the number of rows: 5 1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | import java.util.Scanner; public class Pattern4 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i = 1; i <= rows; i++) { for (int j = 1; j <= i; j++) { System.out.print(j+" "); } System.out.println(); } for (int i = rows-1; i >= 1; i--) { for (int j = 1; j <= i; j++) { System.out.print(j+" "); } System.out.println(); } sc.close(); } } |
Pattern programs in java: Pattern 5
1 2 3 4 5 6 | Enter the number of rows: 5 1 2 2 3 3 3 4 4 4 4 5 5 5 5 5 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | import java.util.Scanner; public class Pattern5 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i = 1; i <= rows; i++) { for (int j = 1; j <= i; j++) { System.out.print(i+" "); } System.out.println(); } sc.close(); } } |
Pattern programs in java: Pattern 6
1 2 3 4 5 6 | Enter the number of rows: 5 5 4 3 2 1 4 3 2 1 3 2 1 2 1 1 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | import java.util.Scanner; public class Pattern6 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i = rows; i >= 1; i--) { for (int j = i; j >= 1; j--) { System.out.print(j+" "); } System.out.println(); } sc.close(); } } |
Pattern programs in java: Pattern 7
1 2 3 4 5 6 7 8 9 10 | Enter the number of rows: 5 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | import java.util.Scanner; public class Pattern7 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i = rows; i >= 1; i--) { for (int j = 1; j <= i; j++) { System.out.print(j+" "); } System.out.println(); } for (int i = 2; i <= rows; i++) { for (int j = 1; j <= i; j++) { System.out.print(j+" "); } System.out.println(); } sc.close(); } } |
Pattern programs in java: Pattern 8
1 2 3 4 5 6 7 8 9 10 | Enter the number of rows: 5 12345 2345 345 45 5 45 345 2345 12345 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | import java.util.Scanner; public class Pattern8 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i = 1; i <= rows; i++) { for (int j = 1; j < i; j++) { System.out.print(" "); } for (int j = i; j <= rows; j++) { System.out.print(j); } System.out.println(); } for (int i = rows-1; i >= 1; i--) { for (int j = 1; j < i; j++) { System.out.print(" "); } for (int j = i; j <= rows; j++) { System.out.print(j); } System.out.println(); } sc.close(); } } |
Pattern programs in java: Pattern 9
1 2 3 4 5 6 | Enter the number of rows: 5 1 10 101 1010 10101 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | import java.util.Scanner; public class Pattern9 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i = 1; i <= rows; i++) { for (int j = 1; j <= i; j++) { if(j%2 == 0) { System.out.print(0); } else { System.out.print(1); } } System.out.println(); } sc.close(); } } |
Pattern programs in java: Pattern 10
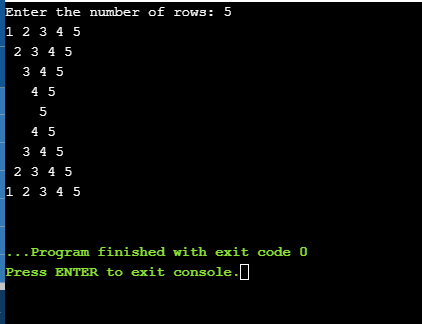
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | import java.util.Scanner; public class pattern10 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows = sc.nextInt(); for (int i = 1; i <= rows; i++) { for (int j = 1; j < i; j++) { System.out.print(" "); } for (int j = i; j <= rows; j++) { System.out.print(j+" "); } System.out.println(); } for (int i = rows-1; i >= 1; i--) { for (int j = 1; j < i; j++) { System.out.print(" "); } for (int j = i; j <= rows; j++) { System.out.print(j+" "); } System.out.println(); } sc.close(); } } |
Pattern programs in java: Pattern 11
1 2 3 4 | 1 2 3 4 5 6 7 8 9 10 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | public class Pattern11 { public static void main(String[] args) { int rows = 4, num = 1; for(int i = 1; i <= rows; i++) { for(int j = 1; j <= i; j++) { System.out.print(num + " "); ++num; } System.out.println(); } } } |
Pattern programs in java: Pattern 12
1 2 3 4 5 6 | Pyramid 1 2 2 3 3 3 4 4 4 4 5 5 5 5 5 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | //Pattern with numbers public class Pattern9 { public static void main(String[] args) { int rows = 5, count = 1; System.out.println("Pyramid"); for (int i = rows; i > 0; i--) { for (int j = 1; j <= i; j++) { System.out.print(" "); } for (int j = 1; j <= count; j++) { System.out.print(count+" "); } System.out.println(); count++; } } } |
Pattern programs in java: Pattern 13
1 2 3 4 5 6 7 8 | Pyramid 1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 1 2 3 4 5 6 1 2 3 4 5 6 7 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | //Pattern with numbers public class Pattern10 { public static void main(String[] args) { int rows = 7, count = 1; System.out.println("Pyramid"); for (int i = rows; i > 0; i--) { for (int j = 1; j <= i; j++) { System.out.print(" "); } for (int j = 1; j <= count; j++) { System.out.print(j+" "); } System.out.println(); count++; } } } |