This post covers the Java Program to swap two numbers without using the third variable. It is easy to swap two numbers using the third variable, we assigned one to a temporary(temp) variable and swap with others.
Now let us see without using the third variable.
Explanation:
With the help of a Scanner class, we take the user input for two integers (a = 5, b = 3).
Now during the process of swapping, we assigned the value of a
equal to the sum of a and b that is,a = a + b;
(a = 5+3 = 8).
Second, we assigned the value of b equal to the difference between a and b that is,b = a - b
(b= 8 – 3 = 5).
Lastly, we assigned the value of a equal to the difference between a and b that is,a = a - b
(a= 8 – 5 = 3).
Then print those swapped values as shown in the program below.
Java program to swap two numbers without using Third Variable
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | //Swapping of two numbers import java.util.Scanner; class SwapTwoNumbers { public static void main(String args[]) { int a, b; System.out.println("Enter the two numbers, a and b"); Scanner in = new Scanner(System.in); a = in.nextInt(); b = in.nextInt(); System.out.println("Values before Swapping\na = "+a+"\nb = "+b); a = a + b; b = a - b; a = a - b; System.out.println("Values after Swapping without third variable\na = "+a+"\nb = "+b); } } |
Output:
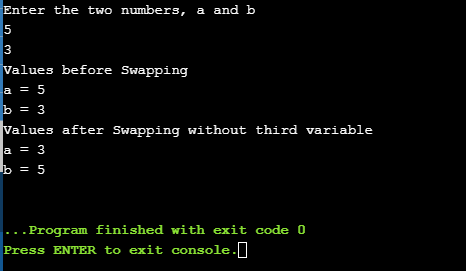