This is an explanation and example for Happy Number in Java. And Let’s begin with an explanation:
Define Happy Number
A number is said to be a happy number if it yields 1 after a few steps and each step is the sum of the squares of the digits of its results. The sum of the squares starts with the given number and till it reaches one.
Follow the diagram below:
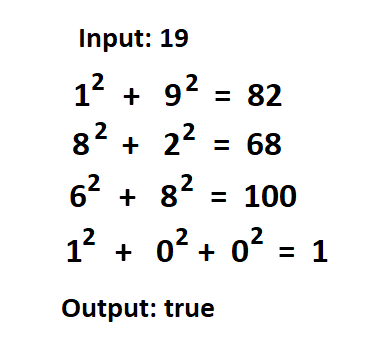
Explanation: In the above diagram input is 19. Then the digits 1 and 9 are squared which gives the result 82. After that, the digits 8 and 2 are squared and yield the results 68 and this continues till the final result is 1. And then we can say that 19 is a happy number, if not then it is not a happy number.
If you do not know how to create a function or if you want to learn about the method, click on the link below.
Java program to check whether the Number is Happy Number or Not:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | import java.util.Scanner; public class CheckHN { //function to check for happyNumber public static int checkForHN(int num) { int temp = 0, sum = 0; //calculation while(num > 0){ temp = num%10; sum = sum + (temp*temp); num = num/10; } return sum; } public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number: "); int num = sc.nextInt(); int result = num; while(result != 1 && result != 4){ result = checkForHN(result); } //check for 1 if(result == 1) System.out.println(num + " is a happy number"); else if(result == 4) System.out.println(num + " is not a happy number"); } } |
Output:
Enter the number: 32
32 is a happy number
Java program to Print Happy Number between 1 to 100:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | import java.util.Scanner; public class Main { //function to check for happyNumber public static int checkForHN(int num) { int temp = 0, sum = 0; //calculation while(num > 0){ temp = num%10; sum = sum + (temp*temp); num = num/10; } return sum; } public static void main(String[] args) { System.out.println("Happy Numbers between 1 to 100"); //checking in a loop for(int i = 1; i <= 100; i++) { int result = i; while(result != 1 && result != 4) { result = checkForHN(result); } //check for 1 if(result == 1){ System.out.print(i + " "); } } } } |
Output:
Happy Numbers between 1 to 100
1 7 10 13 19 23 28 31 32 44 49 68 70 79 82 86 91 94 97 100
Then again, You can print the first 10 Happy Number by changing the loop value to 10 and calling the checkForHN() function.