Reverse a sentence word by word in java:
Reversing a string in java is easy. Here we reverse the sentence of words from back to front rather than reversing each word.
Example: Let us consider a string “I am a code” and the output will be “code a am I“.
Explanation:
First, we take a String from user input with the help of a Scanner class. Then we again with help of split() function we split the input string into separate words that is String[] wordsRev = str.split("\s");
.
Take an empty string to store the reverse sentence. Then the iteration of for loop starts for i equal to the length of the words present in a string. Then the split words are store in the result String from backward inside the for loop.
For loop will continue until i is less than or equal to zero. Integer i will decrease by 1 at every iteration. Since we take the length from backward we decrease the value of i.
At last, print the result String(resultString).
Java Program to Reverse a Sentence Word by Word
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | import java.util.Scanner; public class ReverseTheString { public static void main(String[] args) { Scanner sc = new Scanner(System.in); String str; System.out.println("Enter the String :"); str = sc.nextLine(); //String outputString = reverseTheSentence(str); String[] wordsRev = str.split("\\s"); String resultString = ""; for (int i = wordsRev.length-1; i >= 0; i--) { resultString = resultString + wordsRev[i] + " "; } System.out.println("Output String : "+resultString); sc.close(); } } |
The output of reversing the words in a sentence n java:
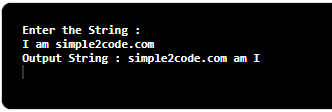