In this tutorial, we will write a program to reverse a string in Java. It means displaying the string from backward.
Consider the string reverse, we write a java program in various ways to reverse it and it will be displayed as esrever. Some of the ways to do it are using:
- for loop
- recursion
- StringBuffer.
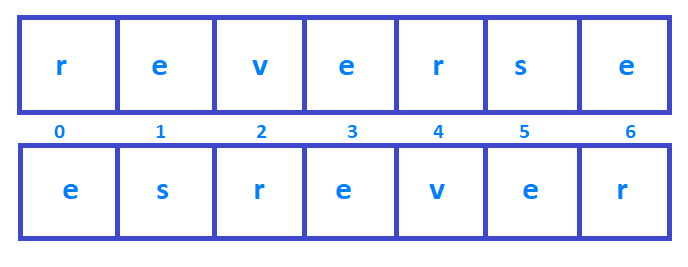
Write a java program to reverse a string:
Using for loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | //reverse a string import java.util.Scanner; class ReversingString { public static void main(String args[]) { String str, revString= ""; Scanner in = new Scanner(System.in); //user input System.out.println("Enter a string: "); str= in.nextLine(); int length = str.length(); for (int i = length - 1 ; i >= 0 ; i--) { revString += str.charAt(i); } //display the reverse result System.out.println("Reverse of the entered string: " + revString); } } |
Output: when the code is is executed it shows the following result.
Enter a string:
I am learning java
Reverse of the entered string: avaj gninrael ma I
Using StringBuffer:
In this method, we use reverse() method of StringBuffer class to reverse a string in java.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | //reverse a string Using StringBuffer import java.util.Scanner; class ReverseOfString { public static void main(String args[]) { String str; Scanner in = new Scanner(System.in); //user input System.out.println("Enter the string: "); str= in.nextLine(); //using StringBuffer StringBuffer sb = new StringBuffer(str); System.out.println("Reverse of entered string is:"+sb.reverse()); } } |
Output:
Enter the string:
reverse
Reverse of entered string is: esrever
Using Recursion:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | //reverse a string using recursion import java.util.Scanner; class Main { public static void main(String args[]) { Main rvrs = new Main(); String str, revString= ""; Scanner in = new Scanner(System.in); //user input System.out.println("Enter a string: "); str= in.nextLine(); String reverse = rvrs.reverseString(str); System.out.println("Reverse of entered string is: "+reverse); } public String reverseString(String str) { if ((null == str) || (str.length() <= 1)) { return str; } return reverseString(str.substring(1)) + str.charAt(0); } } |
Output:
Enter the string:
reverse
Reverse of entered string is: esrever