In this tutorial, we will write a program to display a hollow pattern of stars in java. We will write code for two different shapes patterns. Before that, you may go through the following topic in java.
Hollow rectangle star pattern in java
The program takes a user input for the number of rows and columns for the hollow rectangle. Then using two for loops it will print the star rectangular pattern in java.
Example:
1 2 3 4 5 6 7 8 9 10 | <em>Inputs:</em> <em> Rows: 6 Columns:10</em> <strong>Output:</strong> <strong>********** * * * * * * * * **********</strong> |
Java Program to print Hollow Rectangle Star Pattern.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | import java.util.Scanner; public class Main { public static void main(String args[]) { int rows, columns, i, j; Scanner sc = new Scanner(System.in); System.out.print("Enter the no. of rows: "); rows = sc.nextInt(); System.out.print("Enter the no. of columns: "); columns = sc.nextInt(); System.out.print("Rectangular star Pattern: \n\n"); for (i = 1; i <= rows; i++) { for (j = 1; j <= columns; j++) { if (i == 1 || i == rows || j == 1 || j == columns) System.out.print("*"); else System.out.print(" "); } System.out.println(); } } } |
Output:
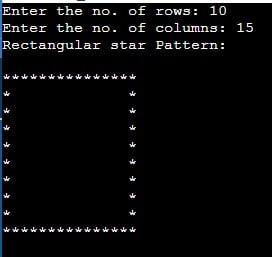
Hollow square star pattern in java
As the square contains only sides and both length and breadth are equal so the program only takes user input for a number of rows and prints the same number of rows and columns.
Example:
1 2 3 4 5 6 7 8 9 10 | <em>Inputs:</em> <em>Rows: 7</em> <strong>Output:</strong> <strong>******* * * * * * * * *</strong> <strong>* * *******</strong> |
Java program to print hollow square pattern of stars
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | import java.util.Scanner; public class Main { public static void main(String[] args) { int rows, i, j; Scanner sc = new Scanner(System.in); System.out.print("Enter the number of rows: "); rows = sc.nextInt(); for (i = 1; i <= rows; i++) { for (j = 1; j <= rows; j++) { if (i == 1 || i == rows || j == 1 || j == rows) System.out.print("*"); else System.out.print(" "); } System.out.println(); } } } |
Output:
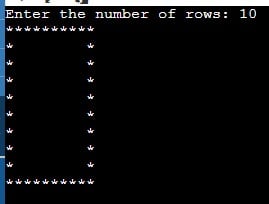