In this tutorial, we will write a java program to convert binary to decimal using while loop. Before that, you must have knowledge of the following topics in java.
Binary number
The binary numbers are based on 0 and 1, so it is a base 2 number. They are the combination of 0 and 1. For example, 1001, 110101, etc.
Decimal Number
These are the numbers with a base of 10, which ranges from 0 to 9. These numbers are formed by the combination of 0 to 9 digits such as 24, 345, etc.
Here is the chart where for the equivalent value of decimal and binary numbers.
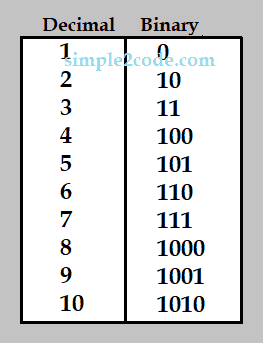
Example:
Input: 101
Output: 5
Input: 111
Output: 7
Now let us go through a program for the conversion of binary to decimal in java using while loop.
Before using a while loop, let us find the conversion of binary number to decimal number by using the parseInt() method of the Integer class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | import java.util.Scanner; public class Main { public static void main(String args[]) { String bin; Scanner sc = new Scanner(System.in); System.out.println("Enter a binary number: "); bin = sc.nextLine(); System.out.println("Equivalent Decimal Value: " + Integer.parseInt(bin, 2)); } } |
Enter a binary number:
1001
Equivalent Decimal Value: 9
Java Program to Convert Binary to Decimal using while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | import java.util.Scanner; public class Main { public static void main(String args[]) { int bin, decimal = 0, i = 0; Scanner sc = new Scanner(System.in); System.out.println("Enter a binary number: "); bin = sc.nextInt(); while (bin != 0) { decimal += ((bin % 10) *Math.pow(2, i)); bin /= 10; i++; } System.out.println("Equivalent Decimal Value: " + decimal); } } |
Output:
Enter a binary number:
1010
Equivalent Decimal Value: 10