Generating Random numbers in java is quite easy as it provides various classes to do that. It comes in handy when needed to apply to any kind of application development that might require random number generation. Let start by knowing random numbers.
What is Randon Number?
We can define Random as something that happens without any conscious decision. Similarly, a random number is a number that is chosen unpredictably or randomly from the set of numbers.
Random Number can be generated using two ways.
- java.util.Random class
- Math.random() method
We will also learn to generate between the range, at last.
Java Program to generate Random Number
1. Using java.util.Random class:
java.util.Random class allows us to generate random numbers of type integers, doubles, floats, longs, and booleans. In the example below, we will see to generate numbers of type Integers and booleans. Then you can apply to other data-types.
Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | import java.util.Scanner; import java.util.Random; public class JavaRandomNumber { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //user Input System.out.println("How many random Numbers do you want?"); int num = sc.nextInt(); Random random = new Random(); //Interger Random Numbers System.out.println(num + " Random Integers are:"); for (int i = 0; i < num; i++) { System.out.println(+random.nextInt()); } System.out.println("\n"); //Booleans Random Numbers System.out.println(num + " Random Booleans are:"); for (int i = 0; i < num; i++) { System.out.println(random.nextBoolean()); } } } |
The output of generating random numbers using java.util.Random class:
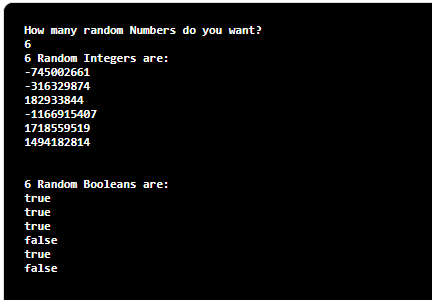
2. Using Math.random() method:
Math.random() method allows you to create only a random number of type doubles as shown below.
Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | import java.util.Scanner; public class JavaRandomNumber { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //user Input System.out.println("How many random Numbers do you want?"); int num = sc.nextInt(); //Doubles Random Numbers System.out.println(num+" Random Doubles are:"); for(int i = 0; i < num; i++) { System.out.println(+Math.random()); } } } |
The output of generating random numbers using Math.random() method:
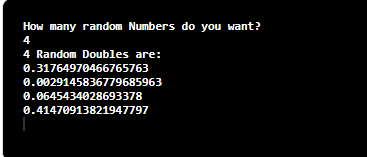
Java Program to generate Random Number within the range
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | import java.util.Scanner; import java.util.Random; public class JavaRandomNumber { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //user Inputs System.out.println("How many random Numbers do you want?"); int num = sc.nextInt(); System.out.println("What is the last range of number starting from 0 to _?"); int lastNum = sc.nextInt(); Random random = new Random(); //Doubles Random Numbers System.out.println("\n"+num+" Random integers within 0 to "+lastNum+ " are : "); for (int i = 0; i < 5; i++) { System.out.println(+random.nextInt(lastNum)); } } } |
The output to generate random numbers between the range in java:
How many random Numbers do you want?
4
What is the last range of number starting from 0 to _?
30
4 Random integers within 0 to 30 are :
1
15
9
28
4
This random generation of numbers within range can be applied to Math.random() method, we only need to replace the code inside the for loop to System.out.println((int)(Math.random() * lastNum));
.