In this tutorial, we will learn how to implement Bubble Sort in java. First, we will start by understanding the Bubble Sort algorithm.
Bubble Sort Algorithm
Bubble sorting is the simplest sorting algorithm that works by comparing two adjacent elements in an array and swapping them if found in the wrong order.
Bubble sort compares the first element with the next one and if found in the wrong order then that compared element in an array are swapped. This algorithm traverse through the entire element in an array.
Time Complexity of Bubble Sort:
- Best case: O(n)
- Average case: O(n^2)
- Worst case: O(n^2)
Bubble Sort in Java program:
We will see the two examples on Bubble sorting in java, one defining the elements in the program and another by taking user input.
Before we begin, you need to have an idea on following:
1. Defining the elements in a program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | public class BubbleSortExample { static void bubbleSort(int[] arr) { int n = arr.length; int temp = 0; for (int i = 0; i < n; i++) { for (int j = 1; j < (n - i); j++) { if (arr[j - 1] > arr[j]) { //swap elements temp = arr[j - 1]; arr[j - 1] = arr[j]; arr[j] = temp; } } } } public static void main(String[] args) { //Defining array elements int arr[] = { 4, 76, 34, 2, 132, 16, 1 }; System.out.println("Order of elements before Bubble Sort"); for (int i = 0; i < arr.length; i++) { System.out.print(arr[i] + " "); } System.out.println(); //sorting array bubbleSort(arr); System.out.println("Order of elements after Bubble Sort"); for (int i = 0; i < arr.length; i++) { System.out.print(arr[i] + " "); } } } |
The Output of Bubble Sort in Java.
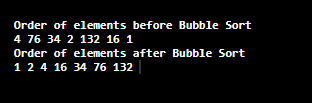
2. By User-Input.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 | import java.util.Scanner; public class BubbleSortExample { static void bubbleSort(int[] arr) { int n = arr.length; int temp = 0; for (int i = 0; i < n; i++) { for (int j = 1; j < (n - i); j++) { if (arr[j - 1] > arr[j]) { //swap elements temp = arr[j - 1]; arr[j - 1] = arr[j]; arr[j] = temp; } } } } public static void main(String[] args) { int n, c; Scanner in = new Scanner(System.in); System.out.println("Enter the no. of elements to be sorted"); n = in .nextInt(); int arr[] = new int[n]; System.out.println("Enter " + n + " integers"); for (c = 0; c < n; c++) arr[c] = in .nextInt(); System.out.println("Order of elements before Bubble Sort"); for (int i = 0; i < arr.length; i++) { System.out.print(arr[i] + " "); } System.out.println(); //sorting array bubbleSort(arr); System.out.println("Order of elements after Bubble Sort"); for (int i = 0; i < arr.length; i++) { System.out.print(arr[i] + " "); } } } |
The Output of the Bubble sorting algorithm in java.
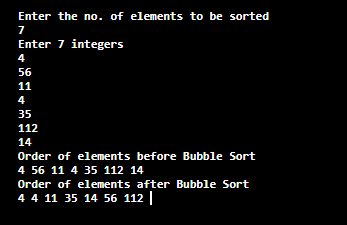