In this tutorial, you will learn about the Automorphic Number along with an example and also code java to check whether the number is automorphic or not.
What is an Automorphic number?
A number is said to be an automorphic number if the square of the given number ends with the same digits as the number itself. Example: 25, 76, 376, etc.
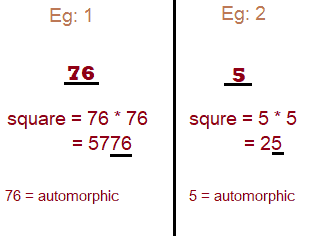
Check Whether a number is an Automorphic Number in Java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | import java.util.Scanner; public class Automorphic { public static void main(String args[]) { Scanner in = new Scanner(System.in); System.out.println("Enter the Number: "); int num = in .nextInt(); int count = 0, sqr = num * num; int temp = num; //copying num into temp //condition applied while (temp > 0) { count++; temp = temp / 10; } int lastSquareDigits = (int)(sqr % (Math.pow(10, count))); //comparing to check Automorphic if (num == lastSquareDigits) System.out.println(num + " is an Automorphic number"); else System.out.println(num + " is not an Automorphic number"); } } |
Output:
Enter the Number:
25
25 is an Automorphic number
//Another execution
Enter the Number:
23
23 is not an Automorphic number
Java Program to Find all Automorphic Numbers in the Interval
Now what if we need to find Automorphic Numbers between the range of a given number, the above example will not work as it is applied only for a single number.
To check all the numbers within some given range of numbers, we will create a separate function that will return a true or false value. In the main function, we will call the created function in a loop so that it can check/call for every number.
The loop will run from the starting number to the range mentioned in the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | import java.util.Scanner; public class AutomorphicClass { public static void main(String args[]) { Scanner in = new Scanner(System.in); int lower, upper; System.out.println("Enter the lower Range: "); lower = in .nextInt(); System.out.println("Enter the Upper Range: "); upper = in .nextInt(); System.out.println("Automorphic numbers between " + lower + " and " + upper); for (int i = lower; i <= upper; i++) { if (checkForAutomorphic(i)) System.out.print(i + " "); } } //funcion private static boolean checkForAutomorphic(int num) { int count = 0, sqr = num * num; int temp = num; //copying num //condition check while (temp > 0) { count++; temp = temp / 10; } int lastSquareDigits = (int)(sqr % (Math.pow(10, count))); return num == lastSquareDigits; } } |
Output:
Enter the lower Range:
1
Enter the Upper Range:
100
Automorphic numbers between 1 and 100
1 5 6 25 76