As we know from the above loop that if the condition is found false then the loop will not be executed but what if we want the loop to execute once (like the menu drive program) even if the condition is found to be false. So in this kind of situation do-while loop comes into play.
It is the same as the while loop where the loop is terminated on the basis of the test condition. The main difference is that the do-while loop checks the condition at the end of the loop which allows the do-while to execute the loop at least once.
do-while Flowchart:
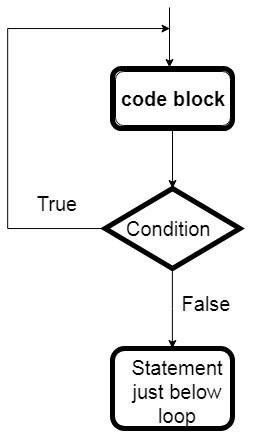
The syntax for do-while loop
in C#:
1 2 3 | do{ //statements.. }while (condition); |
You should use do while loop if the code needs to be executed at least once.
Example of C# do-while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | using System; namespace Loops { class DoWhileProgram { static void Main(string[] args) { int i = 1; //do loop execution do { Console.WriteLine("value of i: {0}", i); i += 1; } while (i <= 10); } } } |
Output:
1 2 3 4 5 6 7 8 9 10 | value of i: 1 value of i: 2 value of i: 3 value of i: 4 value of i: 5 value of i: 6 value of i: 7 value of i: 8 value of i: 9 value of i: 10 |