In this tutorial, we will write a C# program to reverse an array, we have used while loop and for loop to reverse an array in C#.
Question:
Write a C# Program to Reverse an array without using function.
C# Program to Reverse an Array
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | using System; using System.Collections; using System.Collections.Generic; using System.Linq; class ArrayReversing { static void Main(string[] s) { const int EXIT_VALUE = -999; List<int> numbers = new List<int> (); Console.WriteLine("Enter the number and press -999 at the end:"); while (true) { var input = Console.ReadLine(); int i = int.Parse(input); if (i == EXIT_VALUE) break; numbers.Add(i); } int[] array = numbers.ToArray(); Console.WriteLine("Reversed Array is:"); for (int i = array.Length - 1; i > -1; i--) { Console.WriteLine(array[i].ToString()); } } } |
The output of reversing an Array in C#.
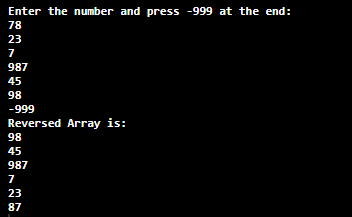