Inheritance is one of the most important concepts of object-oriented programming. In C++, inheritance is the process of deriving the properties and behaviors of one class to another class. It is like a child and parent where the child possesses the properties of the parent.
- The class which inherits the members of another class is called the derived class (child/sub).
- The class whose members are inherited is called the base class (super/parent).
Importance of Inheritance:
- For Code Reusability.
- For Method Overriding (to achieve runtime polymorphism).
A basic example of Inheritance.
1 2 3 4 5 6 7 8 9 10 | class Animal { // eat() function // sleep() function }; class Cat: public Animal { // sound() function }; |
As you can see in the above example Cat class inherits from the Animal class which means that the Cat class has can access the eat() and sleep() methods of Animal and also has its own additional method sound().
Modes of Inheritance.
It is the visibility mode that is used while deriving a parent class. such as:
1 2 3 4 | class derived_class_name : visibility-mode parent_class_name { // derived class members. } |
We can derive the parent class publicly or privately.
Public mode: When the derive class publicly inherits the base class then the public members of the base class also become the public members of the derived class. Therefore, the public members of the base class are accessible by the objects of the derived class as well as by the member functions of the base class.
Private mode: When the derive class privately inherits the base class then the public members of the base class become the private members of the derived class.
Types Of Inheritance
C++ supports the following five types of inheritance.
- Single inheritance
- Multiple inheritance
- Multilevel inheritance
- Hierarchical inheritance
- Hybrid inheritance
1. Single Inheritance
It refers to an inheritance where a child inherits a single-parent class.
In the diagram below, class B inherits class A.
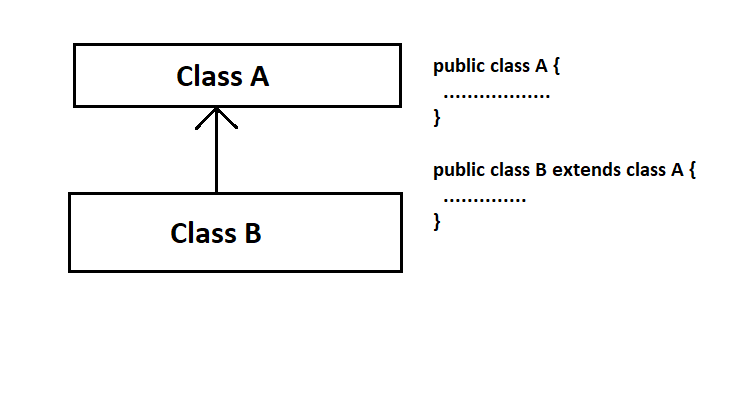
Syntax:
1 2 3 4 | class subclass_name : access_mode base_class_name { //body of subclass }; |
Example: C++ example for single inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | #include <iostream> using namespace std; class One { public: int num1 = 10; int num2 = 20; int add() { int sum = num1 + num2; return sum; } }; class Two : public One { public: void print() { //Inheriting method int result = add(); cout <<"Result of addition: "<< result << endl; } }; int main() { Two two; //Inheriting Field cout << two.num1 << endl; two.print(); return 0; } |
Output:
10
Result of addition: 30
2. Multiple Inheritance
Multiple inheritance is the process where the single class inherits the properties from two or more classes.
In the diagram below, class C inherits from both Class A and Class B.
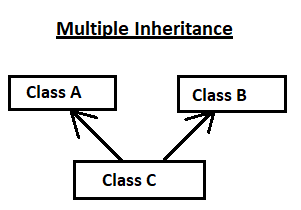
Syntax:
1 2 3 4 | class subclass_name : specifier base_class1, specifier base_class2, .... { //body of subclass }; |
Example: C++ example for multiple inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | #include <iostream> using namespace std; class One { protected: int num1; public: void getNum1(int n) { num1 = n; } }; class Two { protected: int num2; public: void getNum2(int n) { num2 = n; } }; //Inherits from One and Two class Three : public One, public Two { public: void print() { int sum = num1 + num2; cout << "num1 : " << num1 << endl; cout << "num2 : " << num2 << endl; cout << "Result of sum: " << sum; } }; int main() { Three th; th.getNum1(5); th.getNum2(10); th.print(); return 0; } |
Output:
num1 : 5
num2 : 10
Result of sum: 15
3. Multilevel Inheritance
In this type inheritance derived class is created from another derived class. In multilevel inheritance, one class inherits from another class which is further inherited by another class. The last derived class possesses all the members of the above base classes.
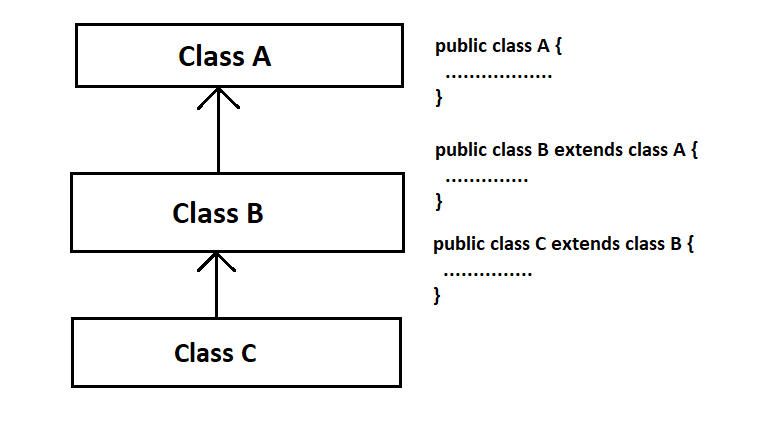
Example: C++ example for multilevel inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | #include <iostream> using namespace std; class Animal { public: void sleep() { cout<<"Animal sleep"<<endl; } }; class Dog: public Animal { public: void bark() { cout<<"Dog Barking"<<endl; } }; class Puppy: public Dog { public: void puppies() { cout<<"Puppies"; } }; int main(void) { Puppy d; d.sleep(); d.bark(); d.puppies(); return 0; } |
Output:
Animal sleep
Dog Barking
Puppies
4. Hierarchical Inheritance
The process of deriving more than one class from a base class is called hierarchical inheritance. In other words, we create more than one derived class from a single base class.
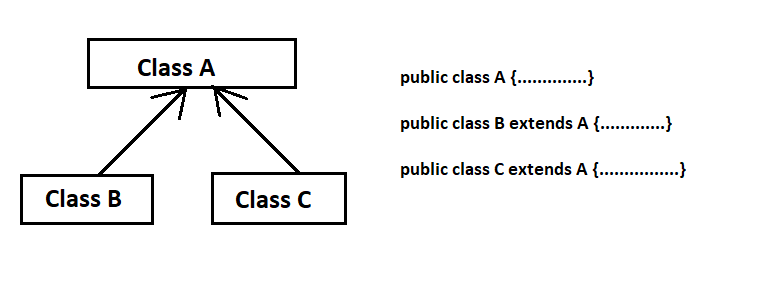
Syntax:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | class One { // Class One members } class Two : public One { // Class Two members. } class Three : public One { // Class Three members } class Four : public One { // Class Four members } |
Example: C++ example for hierarchical Inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | #include <iostream> using namespace std; class Animal { public: void sleep() { cout<<"Animal sleep"<<endl; } }; class Dog: public Animal { public: void dogSound() { cout<< "Barks" <<endl; } }; class Cat: public Animal { public: void catSound() { cout<< "Meow" << endl; } }; int main(void) { Cat c; c.sleep(); c.catSound(); Dog d; d.sleep(); d.dogSound(); return 0; } |
Output:
Animal sleep
Meow Animal sleep
Barks
5. Hybrid (Virtual) Inheritance
Hybrid inheritance is the combination of more than one type of inheritance. In the diagram below is the combination of hierarchical Inheritanceand multiple inheritance.
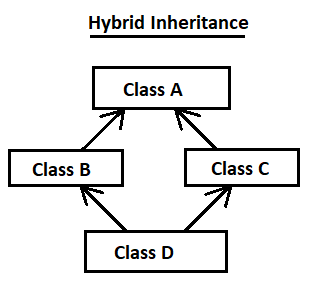
Example: C++ example for hierarchical Inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | #include <iostream> using namespace std; class One { protected: int a = 20; public: void getOne() { cout << "Value of a: " << a <<endl; } }; class Two { protected: int b = 30; public: void getTwo() { cout << "Value of b: " << b <<endl; } }; class Three : public One { protected: int c = 50; public: void getThree() { cout << "Value of c: " << c <<endl; } }; class Four : public Two, public Three { protected: int d; public: void add() { getOne(); getTwo(); getThree(); cout << "Sum : " << a + b + c; } }; int main() { Four fr; fr.add(); return 0; } |
Output:
Value of a: 20
Value of b: 30
Value of c: 50
Sum : 100
Ambiguity in Inheritance
When there is a function present with the same name in two or more base classes then during multiple inheritances or single inheritance an ambiguity can occur in such cases.
Example: C++ example for ambiguity in multiple inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | #include <iostream> using namespace std; class One { public: void display() { cout << "Class One" << endl; } }; class Two { public: void display() { cout << "Class Two" << endl; } }; class Three : public One, public Two { public: void print() { display(); } }; int main() { Three th; th.print(); return 0; } |
There are display() functions in both the classes of One and Two. The following error will be displayed after execution.
1 2 | error: reference to ‘display’ is ambiguous display(); |
To overcome this problem we need to do the following changes in Class Three by using the class resolution operator (::).
1 2 3 4 5 6 7 8 9 | class Three : public One, public Two { public: void print() { One :: display(); Two :: display(); } }; |