break statement terminates the loop and transfers the execution process immediately to a statement following the loop.
break statement is mostly used in a switch statement to terminate the cases present in a switch statement.
The use of break statements in nested loops terminates the inner loop and the control is transferred to the outer loop.
break statement Flowchart:
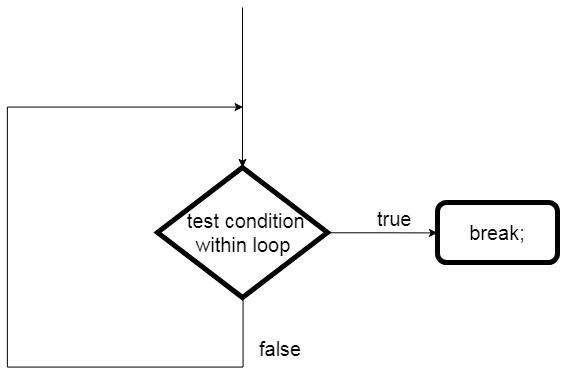
Syntax of break
statement in C++:
1 | break; |
Example on C++ break Statement
Example: C++ program to demonstrate the use of break statement in single loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | #include <iostream> using namespace std; int main() { for (int i = 1; i <= 10; i++) { if (i == 7) { //when i becomes 7 then it will come out of for loop break; } cout << "i is: " << i << endl; } } |
Output:
1 2 3 4 5 6 | i is: 1 i is: 2 i is: 3 i is: 4 i is: 5 i is: 6 |
Example: C++ program for break statement used in nested loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | //using break statement inside nested loop #include <iostream> using namespace std; int main() { // outer loop for (int i = 1; i <= 5; i++) { //inner loop for (int j = 1; j <= 2; j++) { if (i == 4) { //if i becomes 4, it will come out of inner loop break; } cout << "i = " << i << ", j = " << j << endl; } } return 0; } |
Output:
1 2 3 4 5 6 7 8 | i = 1, j = 1 i = 1, j = 2 i = 2, j = 1 i = 2, j = 2 i = 3, j = 1 i = 3, j = 2 i = 5, j = 1 i = 5, j = 2 |
In the above program, for the value i = 4
is skipped because when we reached i
value as four, break statement is applied the execution came out of the inner loop, skipping the inner loop for 4.