In this tutorial, we will learn to convert a decimal number into binary in C++. Before that, you must have knowledge of the following topics in C++.
Binary number
The binary numbers are based on 0 and 1, so it is a base 2 number. They are the combination of 0 and 1. For example, 1001, 110101, etc.
Decimal Number
These are the numbers with a base 10, which ranges from 0 to 9. These numbers are formed with the combination of 0 to 9 digits such as 24, 345, etc.
Here is the chart where for the equivalent value of decimal and binary numbers.
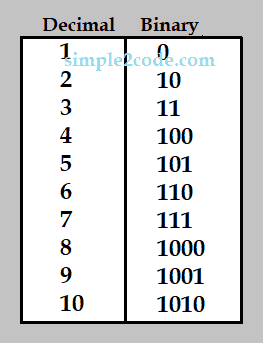
Now let us go through a program for the conversion of decimal to binary in C++.
C++ Program to Convert Decimal to Binary
The program asks the user to enter a decimal number and using a for loop it calculates the binary value. Lastly, display the equivalent binary value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | #include <iostream> using namespace std; int main() { int bin[10], deci, i; cout << "Enter the decimal number: "; cin >> deci; for (i = 0; deci > 0; i++) { bin[i] = deci % 2; deci = deci / 2; } //Display the converted decimal number cout << "Binary value: "; for (i = i - 1; i >= 0; i--) cout << bin[i]; return 0; } |
Output:
Enter the decimal number: 10
Binary value: 1010
Also, check out a similar program: