In this article, we will write a C++ Program for Automorphic Number. The following C++ programming topic is used in the program below.
A number is said to be an automorphic number if the square of the given number ends with the same digits as the number itself. Example: 25, 76, 376, etc.
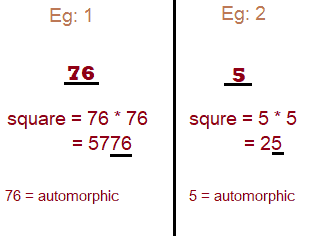
Automorphic Number Program in C++
The C++ program to check automorphic number below takes the number from the user as input and checks accordingly.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | #include <iostream> using namespace std; int main() { int num, flag = 0, sqr; //user input cout << "Enter the number: "; cin >> num; sqr = num * num; int temp = num; //calculation while (temp > 0) { if (temp % 10 != sqr % 10) { flag = 1; break; } temp /= 10; sqr /= 10; } if (flag == 1) cout << num << ": Not an Automorphic number."; else cout << num << ": Automorphic number."; return 0; } |
Output:
Enter the number: 25
25: Automorphic number.
//second execution
Enter the number: 95
95: Not an Automorphic number.