In this tutorial we will learn about Null Pointer, Void Pointer, Wild Pointer and more. If you want to know more on Pointers, click the link given below.
There are eight different types of pointers, some of which we will learn here:
- Null pointer
- Void pointer
- Wild pointer
- Dangling pointer
- Complex pointer
- Near pointer
- Far pointer
- Huge pointer
NULL Pointer
When a pointer is assigned with a null value at the time declaration then it is called a NULL pointer. We use this value when we do not assign any address to the pointer. The null pointer always contains a constant value of 0.
C example to illustrate the use of NULL pointer:
1 2 3 4 5 6 7 8 9 | #include <stdio.h> int main() { int *ptr = NULL; //null pointer printf(“The value inside ptr: %x”, ptr); return 0; } |
Output:
1 | The value inside ptr: 0 |
Void Pointer
A void pointer is also called a generic pointer. This type of pointer can store the address of any variable no matter the data type. It is declared by the keyword void
.
We have learned that the variable whose address is stored in the pointer must be of the same data type that of the pointer. For example: if we declare a pointer with int
type then it cannot points to float type or any other type variable except int. So to avoid this problem void pointer is used. With the use of void, we can point the pointer to any variable with any of the data types without having to typecast.
But if you try to print the value of the variable to which the void pointer is pointing in the program directly then you will get a compile-time error: invalid use of void expression
. So while printing the value, we need to typecast the void pointer to the required data type. We will illustrate it in a program below.
Syntax and declaration of void pointer:
1 2 3 4 | void *pointer_name; //Declaration void *ptr; |
C example to illustrate the use of Void pointer:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | #include <stdio.h> int main() { int a = 10; void *ptr; ptr = &a; //address of varibale a printf("The address of variable a: %p\n", ptr); //size of void pointer printf("The size of void pointer is: %d\n", sizeof(ptr)); return 0; } |
Output: We have discussed about the size of a pointer in Pointer Section.
1 2 | The address of variable a: 0x7ffc0fcac04c The size of void pointer is: 8 |
C example of void pointer while printing values.
The following program tries to print the value of the variable to which the pointer pointing. Normally for any other data type, we simply print the value by *pointer_name
. But here, it throws an error.
1 2 3 4 5 6 7 8 9 10 11 12 13 | #include <stdio.h> int main() { int a = 10; void *ptr; ptr = &a; //error while printing the value printf("The value of variable a: %p\n", *ptr); return 0; } |
Output: Following compile-time error is shown.
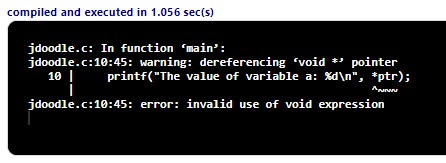
Now to overcome this error and actually display the value of the variable, we will typecast the pointer while printing to remove the error as shown in the code below.
1 2 3 4 5 6 7 8 9 10 11 12 13 | #include <stdio.h> int main() { int a = 10; void *ptr; ptr = &a; //typecasting is performed on pointer printf("The value of variable a: %d\n", *(int*)ptr); return 0; } |
Output:
1 | The value of variable a: 10 |
Wild Pointers
If a pointer is not initialized with a valid address or to anything then the pointer is called Wild Pointer. And because it is not initialized, it may be pointing to some unknown memory location which may cause a problem in the program.
1 2 3 4 5 6 7 8 9 10 | #include <stdio.h> int main() { //wild pointer int *ptr; printf("\n%d", *ptr); return 0; } |
Output:
1 | bash: line 1: 8 Segmentation fault (core dumped) |
Dangling Pointer
If a pointer points to the memory location that has been already deleted is called Dangling Pointer.
Dangling pointers mostly occurs when we are deleting or de-allocating an object (to which one of the pointers was pointing) without modifying the pointer value in the program. That means the pointer is still pointing to that deleted or de-allocated memory and those pointers are referred to as wild/dangling pointers. It causes the segmentation faults
There are three different situation where dangling pointer can occur. You need to use free()
function to de-allocate the memory.
Example 1: De-allocation of memory
1 2 3 4 5 6 7 8 9 10 11 | #include<stdlib.h> int main() { int *ptr = (int *)malloc(sizeof(int)); free(ptr);//Dangling pointer //points to NULL ptr = NULL; } |
Example 2: Function call
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | #include<stdio.h> int *func() { int y = 25; return &y; } int main() { int *ptr = func(); fflush(stdin); // ptr points to something which is not valid printf("The value of y: %d", *ptr); return 0; } |
The above program will through a segmentation fault. The problem will not appear if y is declared static. The dangling will not appear if the pointer is pointed to the static variable.
In the example below, we use the y
as static.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | #include<stdio.h> int *func() { static int y = 25; return &y; } int main() { int *ptr = func(); fflush(stdin); //Not dangling pointer printf("The value of y: %d", *ptr); return 0; } |
Output:
1 | The value of y: 25 |
Example 3: Variable goes out of scope
Here the pointer used is dangling pointer because of the scope. See the syntax below.
1 2 3 4 5 6 7 8 9 10 11 12 13 | void main() { int *ptr; //statement { int ch; ptr = &ch; } //statement } |