Nested Structure in C is nothing but the structure within a structure. We can declare a structure inside a structure and have its own member function. With the help of nested structure, complex data types are created.
The struct declare inside another struct has its own struct variable to access the data members of its own. Following is the Syntax of nested structure.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | structure S_name1 { data_type member1; data_type member2; data_type member3; ... structure S_name2 { data_type member_1; data_type member_2; data_type member_3; ... }, var1 } var2; |
Structure can be nested in two different ways:
1. By Embedding structure
Embedding method means to declare structure inside a structure. It is written in following way in C.
1 2 3 4 5 6 7 8 9 10 11 12 13 | struct Dept { int D_id; char D_name[30]; struct Emp { int emp_name[30]; int emp_id; int emp_add; }e1; //Emp Variable }d1;//Dept variable |
2. By separating structure
This is the second method where the structures are declared separately but the dependent structure is declared inside the main structure as one of its members. And it is written in the following way in C.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | //Dependent structure struct Emp { int emp_name[30]; int emp_id; int emp_add; }; //main Structure struct Dept { int D_id; char D_name[30]; struct Emp e1;//Emp variable }d1; |
Accessing Nested structure in C
Accessing the members is done by (.
) dot operator (already discussed). But in a nested structure, members of the nested structure (inner structure) is accessed through the outer structure followed by the (.
) dot operator.
Consider the above Dept
and Emp
structure, if we want to access the Emp
members, we need to access through Dept
and is done in following way.
d1.e1.emp_name;
d1.e1.emp_id;
d1.e1.emp_add;
Note: You can extended the nesting of structure to any level.
C Program for Nested Structure
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | #include <stdio.h> struct college { int col_id; char col_name[40]; }; struct student { int Stud_id; float score; struct college c1; //college variable }s1; int main() { printf("College Detail\n"); printf("Enter College name: "); scanf("%[^\n]s", s1.c1.col_name); printf("Enter college ID: "); scanf("%d", &s1.c1.col_id); printf("\nStudent Detail\n"); printf("Enter Student ID: "); scanf("%d", &s1.Stud_id); printf("Enter Score: "); scanf("%d", &s1.score); printf("----------DISPLAY-----------\n"); printf("College ID: %d\n", s1.c1.col_id); printf("College Name: %s\n", s1.c1.col_name); printf("\nStudent Id: %d\n", s1.Stud_id); printf("Student Score: %d\n", s1.score); return 0; } |
Output:
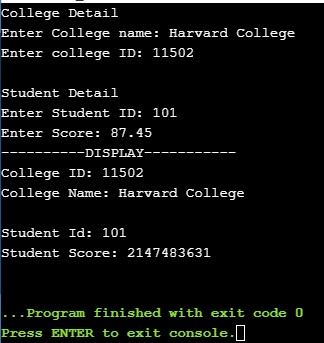