goto statement in c allows the user in the program to jump the execution to the labeled statement inside the function. The label (tag) is used to spot the jump statement.
NOTE: Remember the use of goto is avoided in programming language because it makes difficult to trace the control flow of a program, making the program hard to understand and hard to modify.
goto statement Flowchart:
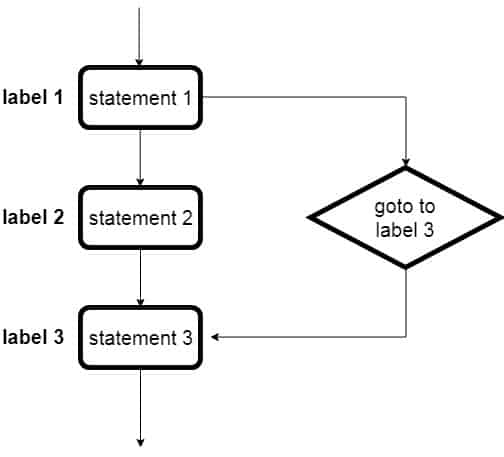
Syntax of goto statement:
1 2 3 4 | goto label; .... .... label: statement; //label to jump |
Example of goto statement in C Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | #include <stdio.h> void main() { int i = 1; label: do { if (i == 8) { //skip the iteration i++; goto label; } printf("Value of i: %d\n", i); i++; } while (i <= 10); } |
The output of continue statement.
1 2 3 4 5 6 7 8 9 | Value of i: 1 Value of i: 2 Value of i: 3 Value of i: 4 Value of i: 5 Value of i: 6 Value of i: 7 Value of i: 9 Value of i: 10 |