In C programming, for loop is a more efficient loop structure and is an entry-control loop. The iteration continues until the stated condition becomes false.
It has three computing steps as shown in the syntax below.
- initialization: The first step is the initialization of the variable and is executed only once. And need to end with a semicolon(;).
- condition: Second is condition check, it checks for a boolean expression. If true then enter the block and if false exit the loop. And need to end with a semicolon(;).
- Increment or Decrement: The third one is increment or decrement of the variable for the next iteration. Here, we need to use the semicolon(;).
for loop Flowchart:
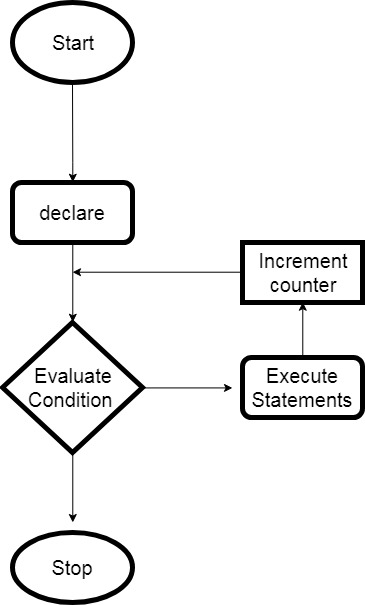
Syntax of for loop.
1 2 3 4 | for(initialization; condition; Increment or Decrement) { // Statements } |
Example of for loop in C Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> int main() { int num; //execution of for loop for (num = 5; num <= 10; num++) { printf("The values of num: %d\n", num); } return 0; } |
The output of for loop in C programming.
The values of num: 5
The values of num: 6
The values of num: 7
The values of num: 8
The values of num: 9
The values of num: 10