In this article, you will learn about the C program to solve Dining Philosophers problem.
The Dining Philosophers Problem:
Let there be 5 philosophers (for example) sitting around a round table for dinner. Each philosopher needs two forks to eat but there are only 5 forks (equal to the number of philosophers around the table).
A philosopher may eat if he/she can pick up two adjacent forks. But the 5 philosophers cannot eat at the same time because they can have only 1 fork on one hand and empty on the other and not able to eat. After that, each of them waits for the adjacent philosopher to finish which leads to a deadlock situation and each of the philosophers starve.
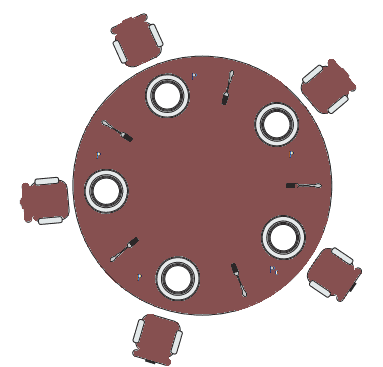
The problem is to create a behaviour (algorithm) for the philosophers such that each philosopher would not starve and able to alternate between eating and thinking.
Program for Dining Philosophers Problem in C
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 | #include <stdio.h> #define n 5 //Number of philosophers around the table int completedPhilosophers = 0, i; struct fork { int taken; } ForkAvail[n]; struct philosp { int left; int right; } Philostatus[n]; void goForDinner(int philID) { //same like threads concept here cases implemented if (Philostatus[philID].left == 10 && Philostatus[philID].right == 10) printf("Philosopher %d completed his dinner\n", philID + 1); //if already completed dinner else if (Philostatus[philID].left == 1 && Philostatus[philID].right == 1) { //if just taken two forks printf("Philosopher %d completed his dinner\n", philID + 1); Philostatus[philID].left = Philostatus[philID].right = 10; //remembering that he completed dinner by assigning value 10 int otherFork = philID - 1; if (otherFork == -1) otherFork = (n - 1); ForkAvail[philID].taken = ForkAvail[otherFork].taken = 0; //releasing forks printf("Philosopher %d released fork %d and fork %d\n", philID + 1, philID + 1, otherFork + 1); completedPhilosophers++; } else if (Philostatus[philID].left == 1 && Philostatus[philID].right == 0) { //left already taken, trying for right fork if (philID == (n - 1)) { if (ForkAvail[philID].taken == 0) { //KEY POINT OF THIS PROBLEM, THAT LAST PHILOSOPHER TRYING IN reverse DIRECTION ForkAvail[philID].taken = Philostatus[philID].right = 1; printf("Fork %d taken by philosopher %d\n", philID + 1, philID + 1); } else { printf("Philosopher %d is waiting for fork %d\n", philID + 1, philID + 1); } } else { //except last philosopher case int dupphilID = philID; philID -= 1; if (philID == -1) philID = (n - 1); if (ForkAvail[philID].taken == 0) { ForkAvail[philID].taken = Philostatus[dupphilID].right = 1; printf("Fork %d taken by Philosopher %d\n", philID + 1, dupphilID + 1); } else { printf("Philosopher %d is waiting for Fork %d\n", dupphilID + 1, philID + 1); } } } else if (Philostatus[philID].left == 0) { //nothing taken yet if (philID == (n - 1)) { if (ForkAvail[philID - 1].taken == 0) { //KEY POINT OF THIS PROBLEM, THAT LAST PHILOSOPHER TRYING IN reverse DIRECTION ForkAvail[philID - 1].taken = Philostatus[philID].left = 1; printf("Fork %d taken by philosopher %d\n", philID, philID + 1); } else { printf("Philosopher %d is waiting for fork %d\n", philID + 1, philID); } } else { //except last philosopher case if (ForkAvail[philID].taken == 0) { ForkAvail[philID].taken = Philostatus[philID].left = 1; printf("Fork %d taken by Philosopher %d\n", philID + 1, philID + 1); } else { printf("Philosopher %d is waiting for Fork %d\n", philID + 1, philID + 1); } } } else {} } int main() { for (i = 0; i < n; i++) ForkAvail[i].taken = Philostatus[i].left = Philostatus[i].right = 0; while (completedPhilosophers < n) { /* Actually problem of deadlock occur only they try to take at same time This for loop will say that they are trying at same time. And remaining status will print by go for dinner function */ for (i = 0; i < n; i++) goForDinner(i); printf("\nTill now number of philosophers who completed dinner are: %d", completedPhilosophers); } return 0; } |
The output of the Dining philosopher problem in c programming:
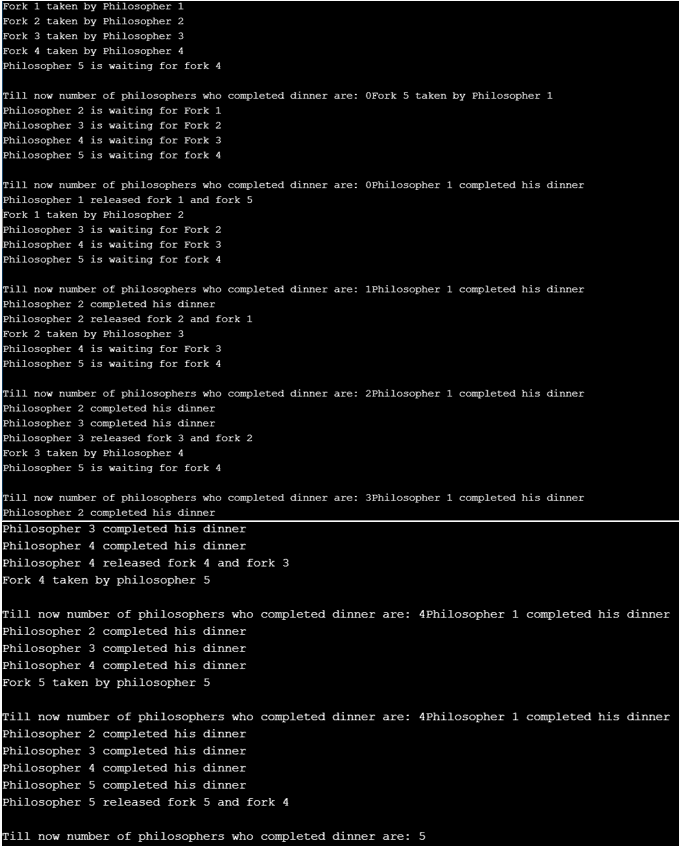